编写程序完成:链表的构建、查找(按位置查找以及按值查找)、插入、删除操作,并实现main程序
时间: 2023-05-29 15:01:17 浏览: 89
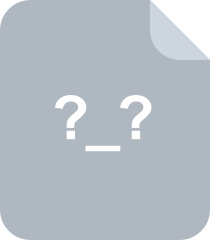
编写完整的程序实现顺序的建立、查找、插入、删除等基本操作
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 数据域
struct Node *next; // 指针域
} Node;
// 链表初始化
Node* initList() {
Node *head = (Node*)malloc(sizeof(Node)); // 创建头结点
head->next = NULL; // 头结点指针域为NULL
return head; // 返回头指针
}
// 链表插入操作
void insert(Node *head, int pos, int value) {
Node *pre = head;
for (int i = 0; i < pos - 1; i++) {
if (pre == NULL) { // 链表长度不够
printf("插入位置不合法!\n");
return;
}
pre = pre->next;
}
Node *newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
newNode->data = value;
newNode->next = pre->next;
pre->next = newNode;
}
// 链表删除操作
void delete(Node *head, int pos) {
Node *pre = head;
for (int i = 0; i < pos - 1; i++) {
if (pre == NULL || pre->next == NULL) { // 链表长度不够
printf("删除位置不合法!\n");
return;
}
pre = pre->next;
}
Node *delNode = pre->next;
pre->next = delNode->next;
free(delNode); // 释放内存
}
// 按值查找节点的位置
int searchByValue(Node *head, int value) {
Node *p = head->next;
int index = 1;
while (p != NULL) {
if (p->data == value) { // 找到节点
return index;
}
p = p->next;
index++;
}
return -1; // 没找到
}
// 按位置查找节点的值
int searchByIndex(Node *head, int pos) {
Node *p = head->next;
for (int i = 0; i < pos - 1; i++) { // 找到该位置的节点
if (p == NULL) { // 链表长度不够
printf("查找位置不合法!\n");
return -1;
}
p = p->next;
}
return p->data; // 返回该节点的值
}
// 遍历链表并输出节点的值
void traverseList(Node *head) {
Node *p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Node *head = initList(); // 初始化链表
insert(head, 1, 10); // 在第1个位置插入10
insert(head, 2, 20); // 在第2个位置插入20
insert(head, 1, 30); // 在第1个位置插入30
traverseList(head); // 输出链表
printf("第%d个位置的节点值为%d\n", 2, searchByIndex(head, 2)); // 查找第2个位置的节点值
printf("元素%d的位置为%d\n", 20, searchByValue(head, 20)); // 查找元素20的位置
delete(head, 3); // 删除第3个位置的节点
traverseList(head); // 输出链表
return 0;
}
阅读全文
相关推荐
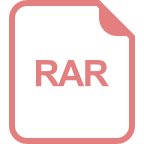

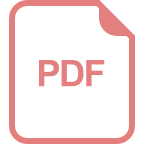
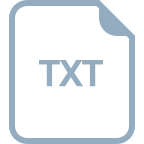
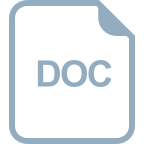
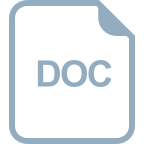
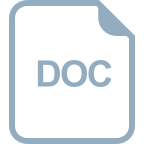
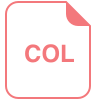
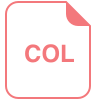
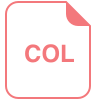
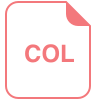



