单链表的构建、查找(按位置查找以及按值查找)、插入、删除操作,并实现main程序。
时间: 2023-04-25 08:05:48 浏览: 64
单链表是一种常见的数据结构,它由一系列节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。单链表的构建、查找、插入和删除操作如下:
1. 构建单链表:从头节点开始,依次插入新节点,直到链表末尾。
2. 按位置查找:从头节点开始,依次遍历链表,直到找到指定位置的节点。
3. 按值查找:从头节点开始,依次遍历链表,直到找到指定值的节点。
4. 插入节点:先找到要插入的位置,然后将新节点插入到该位置之前。
5. 删除节点:先找到要删除的节点,然后将该节点从链表中删除。
下面是一个简单的单链表实现及其main程序:
```c++
#include <iostream>
using namespace std;
// 定义单链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 构建单链表
ListNode* createList(int n) {
ListNode* head = new ListNode(0);
ListNode* p = head;
for (int i = 1; i <= n; i++) {
int x;
cin >> x;
ListNode* node = new ListNode(x);
p->next = node;
p = p->next;
}
return head->next;
}
// 按位置查找
ListNode* findByIndex(ListNode* head, int index) {
if (index < 1) return NULL;
ListNode* p = head;
for (int i = 1; i < index && p != NULL; i++) {
p = p->next;
}
return p;
}
// 按值查找
ListNode* findByValue(ListNode* head, int value) {
ListNode* p = head;
while (p != NULL && p->val != value) {
p = p->next;
}
return p;
}
// 插入节点
void insertNode(ListNode* head, int index, int value) {
ListNode* p = findByIndex(head, index - 1);
if (p == NULL) return;
ListNode* node = new ListNode(value);
node->next = p->next;
p->next = node;
}
// 删除节点
void deleteNode(ListNode* head, int value) {
ListNode* p = head;
while (p->next != NULL && p->next->val != value) {
p = p->next;
}
if (p->next == NULL) return;
ListNode* temp = p->next;
p->next = p->next->next;
delete temp;
}
// 打印单链表
void printList(ListNode* head) {
ListNode* p = head;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
int main() {
int n;
cin >> n;
ListNode* head = createList(n);
printList(head);
int index, value;
cin >> index >> value;
insertNode(head, index, value);
printList(head);
cin >> value;
deleteNode(head, value);
printList(head);
return 0;
}
```
相关推荐
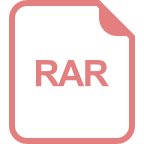
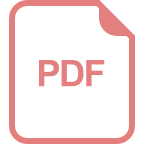
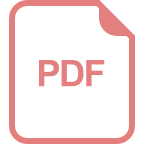














