用C语言编写一组程序,基于单链表,用头插法建表,实现某班学生姓名数据的建表、展示、查找、定位、插入、删除、判定表空,求表长等操作
时间: 2023-10-19 21:21:34 浏览: 48
好的,我可以为您提供有关C语言编写单链表的程序代码。这是一个可以展示、查找、插入、删除和操作表长等功能的程序。
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char name[20];
struct node* next;
} Node;
Node* create_node(char name[20]) {
Node* new_node = (Node*)malloc(sizeof(Node));
if (new_node == NULL) {
printf("Error: unable to allocate memory\n");
exit(1);
}
strcpy(new_node->name, name);
new_node->next = NULL;
return new_node;
}
Node* insert_front(Node* head, char name[20]) {
Node* new_node = create_node(name);
new_node->next = head;
return new_node;
}
int is_empty(Node* head) {
return (head == NULL);
}
int get_length(Node* head) {
int length = 0;
Node* current = head;
while (current != NULL) {
length++;
current = current->next;
}
return length;
}
Node* search(Node* head, char name[20]) {
Node* current = head;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
return current;
}
current = current->next;
}
return NULL;
}
Node* delete(Node* head, char name[20]) {
Node* current = head;
Node* previous = NULL;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
if (previous == NULL) {
head = current->next;
} else {
previous->next = current->next;
}
free(current);
return head;
}
previous = current;
current = current->next;
}
printf("Error: unable to find %s\n", name);
return head;
}
void display(Node* head) {
if (is_empty(head)) {
printf("The list is empty\n");
return;
}
Node* current = head;
printf("The list: ");
while (current != NULL) {
printf("%s ", current->name);
current = current->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
head = insert_front(head, "Alice");
head = insert_front(head, "Bob");
head = insert_front(head, "Charlie");
display(head); // Alice Bob Charlie
Node* search_result = search(head, "Bob");
if (search_result != NULL) {
printf("%s is in the list\n", "Bob");
} else {
printf("%s is not in the list\n", "Bob");
}
head = delete(head, "Bob");
display(head); // Alice Charlie
int length = get_length(head);
printf("The list has %d elements\n", length); // The list has 2 elements
return 0;
}
```
这个程序将创建一个包含学生姓名的单链表,并使用头插法将其构建。它还提供了许多其他功能,例如展示、查找、插入、删除和操作表长等功能。为了保持代码的简洁性,我们只使用了一个结构体节点,存储学生姓名和指向下一个节点的指针。
相关推荐
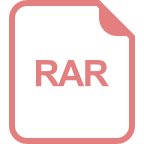
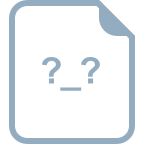
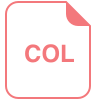
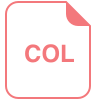
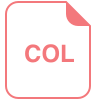
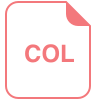
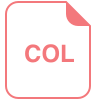









