用C语言写一段程序用于单链表的插入
时间: 2023-12-04 20:01:45 浏览: 27
下面是用C语言写的单链表插入程序:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
struct node *create_list();
void insert_node(struct node *head, int pos, int value);
void print_list(struct node *head);
int main() {
struct node *head = create_list(); // 创建单链表
print_list(head); // 输出单链表的初始状态
insert_node(head, 2, 100); // 在第2个位置插入值为100的节点
print_list(head); // 输出插入节点后的单链表
return 0;
}
// 创建单链表
struct node *create_list() {
struct node *head = (struct node*)malloc(sizeof(struct node));
head->next = NULL;
struct node *tail = head;
int n, value;
printf("请输入单链表的长度:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("请输入第%d个节点的值:", i+1);
scanf("%d", &value);
struct node *p = (struct node*)malloc(sizeof(struct node));
p->data = value;
p->next = NULL;
tail->next = p;
tail = p;
}
return head;
}
// 在单链表的pos位置插入值为value的节点
void insert_node(struct node *head, int pos, int value) {
int i = 0;
struct node *p = head;
while (p != NULL && i < pos-1) {
p = p->next;
i++;
}
if (p == NULL || i > pos-1) {
printf("插入位置不合法!\n");
return;
}
struct node *q = (struct node*)malloc(sizeof(struct node));
q->data = value;
q->next = p->next;
p->next = q;
}
// 输出单链表
void print_list(struct node *head) {
struct node *p = head->next;
printf("单链表的元素为:");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
程序的运行结果如下:
```
请输入单链表的长度:3
请输入第1个节点的值:1
请输入第2个节点的值:2
请输入第3个节点的值:3
单链表的元素为:1 2 3
单链表的元素为:1 2 100 3
```
在上面的程序中,我们定义了一个结构体`node`,用于表示单链表的节点。每个节点包含两个成员变量:`data`表示节点的值,`next`表示指向下一个节点的指针。然后,我们定义了三个函数:
- `create_list()`:用于创建单链表,先创建一个头结点,然后输入单链表的长度和每个节点的值,通过尾插法构建单链表。
- `insert_node(struct node *head, int pos, int value)`:用于在单链表的第`pos`个位置插入值为`value`的节点。首先遍历单链表,找到要插入位置的前一个节点,然后创建一个新节点,将它插入到链表中。
- `print_list(struct node *head)`:用于输出单链表的元素。
在`main`函数中,我们首先创建一个单链表,然后输出它的初始状态。接着,我们在第2个位置插入值为100的节点,再输出插入节点后的单链表。
相关推荐
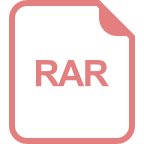
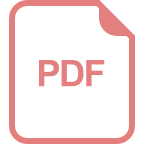
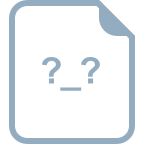














