用C语言写一段定义单链表的代码。
时间: 2023-08-31 10:33:52 浏览: 53
### 回答1:
struct Node
{
int data;
struct Node* next;
};
/* function to create and return a new node */
struct Node *newNode(int data)
{
// Allocate memory for new node
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
// Assign data to this node
node->data = data;
// Initialize next as NULL
node->next = NULL;
return node;
}
### 回答2:
以下是使用C语言编写的一个简单的单链表的定义代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点的结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域
} Node;
// 定义单链表的结构体
typedef struct LinkedList {
Node* head; // 头节点指针
} LinkedList;
// 初始化链表
void initLinkedList(LinkedList* list) {
list->head = NULL;
}
// 在链表末尾添加节点
void addNode(LinkedList* list, int value) {
// 创建新节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
// 如果链表为空,新节点作为头节点
if (list->head == NULL) {
list->head = newNode;
} else {
// 否则遍历找到链表末尾节点并添加新节点
Node* current = list->head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表的所有节点
void printList(LinkedList* list) {
Node* current = list->head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
// 创建链表并初始化
LinkedList list;
initLinkedList(&list);
// 添加节点
addNode(&list, 1);
addNode(&list, 2);
addNode(&list, 3);
addNode(&list, 4);
// 打印链表
printList(&list);
return 0;
}
```
这段代码中,定义了一个单链表的结构体LinkedList,其中包含了一个头节点指针head。每个节点的结构体Node包含一个数据域data和一个指针域next,指针域指向下一个节点。
代码中的initLinkedList函数用于初始化链表,将头节点指针设置为NULL。addNode函数用于在链表末尾添加节点,如果链表为空,则新节点作为头节点;否则遍历链表找到末尾节点并添加新节点。printList函数用于打印链表中所有节点的数据。
在main函数中,创建了一个链表并初始化,然后通过addNode函数添加节点,并使用printList函数打印链表的数据。运行代码会输出链表的数据:1 2 3 4。
### 回答3:
单链表是一种常见的数据结构,它由多个节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。以下是用C语言定义单链表的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构体
typedef struct Node {
int data; // 数据元素
struct Node *next; // 指向下一个节点的指针
} Node;
// 创建单链表节点
Node *createNode(int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = data;
newNode->next = NULL;
}
return newNode;
}
int main() {
// 创建单链表的头节点
Node *head = createNode(0);
// 在头节点之后插入新节点
Node *node1 = createNode(1);
head->next = node1;
// 在链表尾部插入新节点
Node *node2 = createNode(2);
node1->next = node2;
// 遍历并打印单链表中的元素
Node *cur = head;
while (cur != NULL) {
printf("%d ", cur->data);
cur = cur->next;
}
// 释放单链表的内存
cur = head;
while (cur != NULL) {
Node *temp = cur;
cur = cur->next;
free(temp);
}
return 0;
}
```
上述代码中,我们首先定义了单链表的节点结构体,包含了一个整型的数据元素和一个指向下一个节点的指针。然后,我们编写了一个`createNode`函数,用于创建单链表的节点,并返回指向新节点的指针。
在`main`函数中,我们首先创建了单链表的头节点,并将它的值设为0。然后,我们创建了两个新节点,一个插入在头节点之后,一个插入在上一个节点之后,形成一个简单的单链表。最后,我们遍历并打印了单链表中的元素。
最后,我们通过遍历单链表的方式释放了节点所占用的内存,避免内存泄漏。
相关推荐
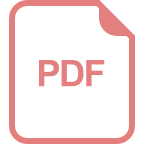
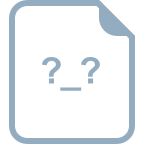
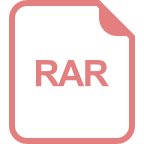














