用c语言1.定义单链表的结点以及指针结构。 2.采用头插入法和尾插入法构建单链表。 3.遍历单链表并输出每个结点的数据值。 4.实现单链表的按位查找和按值查找操作。 5.实现单链表的位插入和删除操作。 6.采用主函数给出运行调用以上单链表操作的接口。
时间: 2024-10-21 09:06:01 浏览: 20
在C语言中,我们通常会通过结构体定义单链表的节点和指针结构:
```c
// 定义单链表节点
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
**1. 首先定义头插入法:**
头插入法会在链表头部添加新的节点:
```c
void insertAtHead(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
printf("Memory allocation failed.");
}
}
```
**2. 然后是尾插入法:**
尾插入法会在链表尾部添加新节点:
```c
void insertAtTail(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
if (*head == NULL) {
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL)
temp = temp->next;
temp->next = newNode;
}
newNode->data = value;
newNode->next = NULL;
} else {
printf("Memory allocation failed.");
}
}
```
**3. 遍历并输出节点数据:**
```c
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
```
**4. 查找操作(位查找和按值查找):**
这里假设链表是有序的,可以实现二分查找:
```c
int binarySearch(Node* head, int value) {
int low = 0, high = getLength(head);
while (low < high) {
int mid = low + (high - low) / 2;
if (head[mid].data < value)
low = mid + 1;
else
high = mid;
}
return (head[low].data == value) ? low : -1;
}
```
**5. 插入和删除操作:**
例如插入位插入和删除:
```c
void insertAtBitPosition(Node** head, int bitIndex, int value) {
// ...实现插入操作...
}
void deleteByValue(Node** head, int value) {
Node* temp = *head, *prev = NULL;
if (temp && temp->data == value) {
*head = temp->next;
free(temp);
return;
}
while (temp && temp->data != value) {
prev = temp;
temp = temp->next;
}
if (temp) {
prev->next = temp->next;
free(temp);
}
}
```
**6. 主函数示例:**
```c
int main() {
Node* head = NULL;
// 初始化链表和调用上述各种操作...
// 示例调用:insertAtHead(&head, 1), insertAtTail(&head, 5), printList(head), etc.
return 0;
}
```
阅读全文
相关推荐
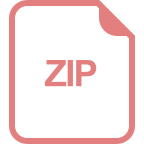
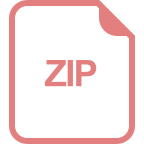

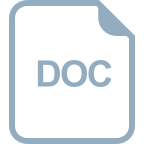













