用delphi语言写一个监控win系统进程的例子
时间: 2023-09-17 08:05:41 浏览: 95
### 回答1:
好的,这是一个使用 Delphi 语言监控 Windows 系统进程的示例程序:
```
uses
Windows, TlHelp32;
function EnumProcesses: string;
var
ContinueLoop: BOOL;
FSnapshotHandle: THandle;
FProcessEntry32: TProcessEntry32;
begin
Result := '';
FSnapshotHandle := CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
FProcessEntry32.dwSize := SizeOf(FProcessEntry32);
ContinueLoop := Process32First(FSnapshotHandle, FProcessEntry32);
while Integer(ContinueLoop) <> 0 do
begin
Result := Result + FProcessEntry32.szExeFile + #13#10;
ContinueLoop := Process32Next(FSnapshotHandle, FProcessEntry32);
end;
CloseHandle(FSnapshotHandle);
end;
begin
ShowMessage(EnumProcesses);
end.
```
这个程序使用了 Windows 库中的 `TlHelp32` 和 `Windows` 单元,通过调用 `CreateToolhelp32Snapshot` 和 `Process32First` 函数获取系统中的进程列表,然后使用 `Process32Next` 函数遍历进程列表,最后使用 `CloseHandle` 函数关闭快照句柄。程序最后使用 `ShowMessage` 函数显示所有进程的名称。
请注意,在使用这个程序之前,你需要在你的程序中包含 `TlHelp32` 和 `Windows` 单元。
### 回答2:
在Delphi中可以使用TProcess类来监控Windows系统进程。下面是一个示例代码:
```
unit MainForm;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs, StdCtrls;
type
TForm1 = class(TForm)
Memo1: TMemo;
Button1: TButton;
Timer1: TTimer;
procedure Button1Click(Sender: TObject);
procedure Timer1Timer(Sender: TObject);
procedure FormClose(Sender: TObject; var Action: TCloseAction);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
uses
ShellApi;
procedure TForm1.Button1Click(Sender: TObject);
begin
Timer1.Enabled := not Timer1.Enabled; // 启动/停止定时器
if Timer1.Enabled then
Button1.Caption := '停止监控'
else
Button1.Caption := '开始监控';
end;
procedure TForm1.Timer1Timer(Sender: TObject);
var
ProcessInfo: TProcessInformation;
ProcessCount: Integer;
i: Integer;
begin
ProcessCount := GetNumberOfProcesses;
Memo1.Clear;
Memo1.Lines.Add('当前进程数量:' + IntToStr(ProcessCount));
Memo1.Lines.Add('');
for i := 0 to ProcessCount - 1 do
begin
// 获取进程id
ProcessInfo.dwProcessId := GetProcessId(i);
// 获取进程句柄
ProcessInfo.hProcess := OpenProcess(PROCESS_QUERY_INFORMATION or PROCESS_TERMINATE, False, ProcessInfo.dwProcessId);
if ProcessInfo.hProcess <> 0 then
begin
try
// 获取进程路径
SetLength(Buffer, MAX_PATH);
if GetProcessImageFileName(ProcessInfo.hProcess, @Buffer[1], MAX_PATH) > 0 then
begin
// 显示进程路径
Memo1.Lines.Add('进程ID:' + IntToStr(ProcessInfo.dwProcessId));
Memo1.Lines.Add('路径:' + Buffer);
Memo1.Lines.Add('');
end;
finally
// 关闭进程句柄
CloseHandle(ProcessInfo.hProcess);
end;
end;
end;
end;
procedure TForm1.FormClose(Sender: TObject; var Action: TCloseAction);
begin
Timer1.Enabled := False; // 关闭时停止定时器
end;
end.
```
这个例子创建了一个Delphi窗体应用程序,其中包含一个Memo组件、一个Button组件和一个Timer组件。当点击按钮时,开启或关闭定时器;定时器超时时,获取当前运行的进程数量和每个进程的路径,并将其显示在Memo控件中。
请注意,此示例代码仅作为参考,需根据实际需要和环境进行适当修改和调整。
### 回答3:
在Delphi中,我们可以使用TProcess类来实现监控Windows系统进程的功能。以下是一个示例代码:
```delphi
uses
Windows, SysUtils, Classes;
type
TProcessMonitor = class
private
FProcessName: string;
FTimer: TTimer;
procedure OnTimer(Sender: TObject);
public
constructor Create(const AProcessName: string);
destructor Destroy; override;
procedure StartMonitoring;
procedure StopMonitoring;
end;
constructor TProcessMonitor.Create(const AProcessName: string);
begin
FProcessName := AProcessName;
FTimer := TTimer.Create(nil);
FTimer.Interval := 1000; // 设置定时器间隔1秒
FTimer.OnTimer := OnTimer;
end;
destructor TProcessMonitor.Destroy;
begin
FTimer.Free;
inherited;
end;
procedure TProcessMonitor.StartMonitoring;
begin
FTimer.Enabled := True; // 启动定时器
end;
procedure TProcessMonitor.StopMonitoring;
begin
FTimer.Enabled := False; // 停止定时器
end;
procedure TProcessMonitor.OnTimer(Sender: TObject);
var
ProcessHandle: THandle;
ProcessId: DWORD;
ProcessFound: Boolean;
ProcessName: array[0..MAX_PATH] of Char;
begin
ProcessFound := False;
ProcessHandle := CreateToolHelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if ProcessHandle <> INVALID_HANDLE_VALUE then
begin
ProcessId := 0;
ProcessName[0] := #0;
if Process32First(ProcessHandle, TProcessEntry32(ProcessName)) then
begin
repeat
if CompareText(ExtractFileName(ProcessName), FProcessName) = 0 then
begin
ProcessFound := True;
ProcessId := TProcessEntry32(ProcessName).th32ProcessID;
Break;
end;
until not Process32Next(ProcessHandle, TProcessEntry32(ProcessName));
end;
CloseHandle(ProcessHandle);
end;
if ProcessFound then
ShowMessage('进程 "' + FProcessName + '" 在运行中,进程ID为: ' + IntToStr(ProcessId))
else
ShowMessage('进程 "' + FProcessName + '" 未找到');
end;
```
使用示例:
```delphi
var
ProcessMonitor: TProcessMonitor;
procedure TForm1.Button1Click(Sender: TObject);
begin
ProcessMonitor := TProcessMonitor.Create('notepad.exe');
ProcessMonitor.StartMonitoring;
end;
procedure TForm1.Button2Click(Sender: TObject);
begin
ProcessMonitor.StopMonitoring;
ProcessMonitor.Free;
end;
```
上述代码中,我们创建了一个TProcessMonitor类来进行进程监控。在构造函数中,我们传入要监控的进程名。StartMonitoring方法启动了一个定时器,在每次触发定时器事件时,通过调用CreateToolHelp32Snapshot方法和Process32First、Process32Next遍历系统进程来查找指定的进程名。如果找到了,就显示进程ID;否则显示未找到的提示信息。使用示例中的代码可以在点击按钮1时开始监控notepad.exe进程,点击按钮2时停止监控。
相关推荐
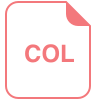













