使用c语言写一个pcm转opus格式的代码
时间: 2023-05-17 20:06:18 浏览: 443
非常感谢您的提问。以下是使用c语言编写pcm转opus格式的代码:
#include <stdio.h>
#include <stdlib.h>
#include <opus/opus.h>
#define FRAME_SIZE 960
#define CHANNELS 1
#define APPLICATION OPUS_APPLICATION_AUDIO
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s input.pcm output.opus\n", argv[0]);
return 1;
}
FILE *input_file = fopen(argv[1], "rb");
if (!input_file) {
printf("Failed to open input file: %s\n", argv[1]);
return 1;
}
FILE *output_file = fopen(argv[2], "wb");
if (!output_file) {
printf("Failed to open output file: %s\n", argv[2]);
fclose(input_file);
return 1;
}
int error;
OpusEncoder *encoder = opus_encoder_create(48000, CHANNELS, APPLICATION, &error);
if (error != OPUS_OK) {
printf("Failed to create encoder: %s\n", opus_strerror(error));
fclose(input_file);
fclose(output_file);
return 1;
}
opus_encoder_ctl(encoder, OPUS_SET_BITRATE(64000));
unsigned char *input_buffer = (unsigned char *) malloc(FRAME_SIZE * CHANNELS * sizeof(short));
unsigned char *output_buffer = (unsigned char *) malloc(FRAME_SIZE * CHANNELS * sizeof(short));
while (1) {
int samples_read = fread(input_buffer, sizeof(short), FRAME_SIZE * CHANNELS, input_file);
if (samples_read <= 0) {
break;
}
int bytes_written = opus_encode(encoder, (opus_int16 *) input_buffer, FRAME_SIZE, output_buffer, FRAME_SIZE * CHANNELS);
if (bytes_written < 0) {
printf("Failed to encode frame: %s\n", opus_strerror(bytes_written));
break;
}
fwrite(output_buffer, 1, bytes_written, output_file);
}
free(input_buffer);
free(output_buffer);
opus_encoder_destroy(encoder);
fclose(input_file);
fclose(output_file);
return 0;
}
阅读全文
相关推荐
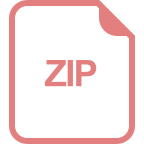
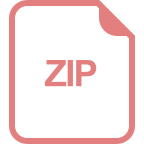
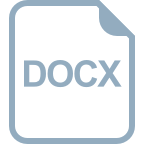

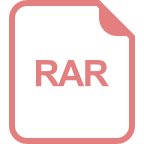
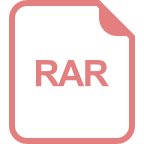
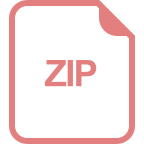
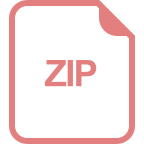
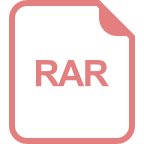
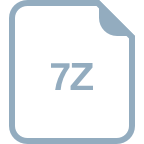
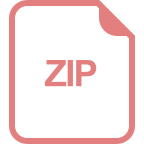
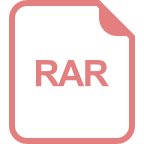
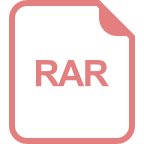
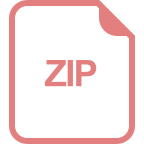

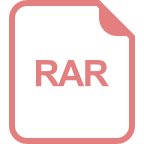
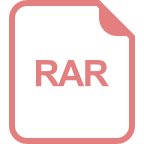