flutter sqlite
时间: 2023-09-03 13:11:56 浏览: 69
Flutter 提供了许多用于本地存储的选项,其中 SQLite 是最常用的选项之一。SQLite 是一个轻量级的关系型数据库管理系统,它可以在移动设备中存储和管理数据。
要在 Flutter 中使用 SQLite,您需要使用 sqflite 插件。该插件提供了 SQLite 的 Dart 版本,可以让您从 Flutter 应用程序中轻松地访问和管理 SQLite 数据库。
以下是使用 sqflite 插件的基本步骤:
1. 添加 sqflite 插件依赖到您的 flutter 项目中
在项目的 pubspec.yaml 文件中添加以下代码
```
dependencies:
sqflite: ^1.3.0
```
2. 导入 sqflite
在您需要使用 sqflite 的文件中添加以下代码
```
import 'package:sqflite/sqflite.dart';
```
3. 打开数据库
使用以下代码打开数据库并创建一个表:
```
Future<Database> database = openDatabase(
// Set the path to the database.
join(await getDatabasesPath(), 'example.db'),
// When the database is first created, create a table to store dogs.
onCreate: (db, version) {
// Run the CREATE TABLE statement on the database.
return db.execute(
"CREATE TABLE dogs(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)",
);
},
// Set the version. This executes the onCreate function and provides a
// path to perform database upgrades and downgrades.
version: 1,
);
```
4. 插入数据
使用以下代码向数据库中插入数据:
```
// Get a reference to the database.
final Database db = await database;
// Insert the Dog into the correct table. You might also specify the
// `conflictAlgorithm` to use in case the same dog is inserted twice.
await db.insert(
'dogs',
dog.toMap(),
conflictAlgorithm: ConflictAlgorithm.replace,
);
```
5. 查询数据
使用以下代码从数据库中查询数据:
```
// Query the table for all The Dogs.
final List<Map<String, dynamic>> maps = await db.query('dogs');
// Convert the List<Map<String, dynamic> into a List<Dog>.
return List.generate(maps.length, (i) {
return Dog(
id: maps[i]['id'],
name: maps[i]['name'],
age: maps[i]['age'],
);
});
```
这些是使用 sqflite 插件进行 SQLite 数据库操作的基本步骤。您可以根据您的需求进行更改和定制。
相关推荐
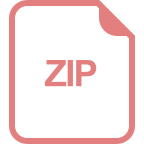
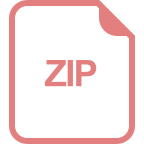














