opengl 转为 shader
时间: 2023-10-07 20:04:36 浏览: 119
OpenGL转换为Shader的过程可以分为以下几个步骤:
1. 定义顶点数据格式:OpenGL中使用顶点数组来存储顶点数据,而Shader中需要定义一个结构体来存储顶点数据,并且需要使用attribute变量来关联顶点数据。
2. 创建Shader程序:OpenGL中使用glCreateProgram函数创建Shader程序,而Shader中需要使用glCreateShader函数创建Shader对象,然后将Shader代码编译成二进制文件,并使用glAttachShader函数将Shader对象附加到程序中。
3. 绑定顶点数据:OpenGL中使用glVertexAttribPointer函数绑定顶点数据,而Shader中需要使用glEnableVertexAttribArray和glVertexAttribPointer函数来绑定顶点数据。
4. 设置Shader参数:OpenGL中使用glUniform函数设置Shader参数,而Shader中需要使用uniform变量来接收参数,并使用glUniform函数将参数传递给Shader程序。
5. 渲染图形:OpenGL中使用glDrawArrays或glDrawElements函数渲染图形,而Shader中需要使用glDrawArrays或glDrawElements函数渲染图形。
需要注意的是,Shader程序的编写需要掌握GLSL语言的基础知识,以及顶点着色器和片段着色器的编写方法。同时,在使用Shader进行渲染时,需要对OpenGL的状态进行管理,例如设置深度测试、启用剪裁面等。
相关问题
QT使用gpu将RAW图转为RGB图,不使用cuda,使用opengl,代码详细
下面是使用OpenGL将RAW图转换为RGB图的代码:
```
#include <QApplication>
#include <QOpenGLWidget>
#include <QOpenGLFunctions>
#include <QOpenGLShaderProgram>
#include <QOpenGLTexture>
#include <QDebug>
#include <iostream>
class RawToRgbWidget : public QOpenGLWidget, protected QOpenGLFunctions
{
public:
RawToRgbWidget(const QString& rawFilePath, QWidget* parent = nullptr)
: QOpenGLWidget(parent),
m_rawFilePath(rawFilePath)
{}
~RawToRgbWidget()
{
makeCurrent();
m_texture.reset();
m_shaderProgram.reset();
doneCurrent();
}
protected:
void initializeGL() override
{
initializeOpenGLFunctions();
// Load raw file
FILE* f = fopen(m_rawFilePath.toLocal8Bit().data(), "rb");
if (!f) {
qCritical() << "Failed to open raw file:" << m_rawFilePath;
return;
}
int width = 1920;
int height = 1080;
int channels = 1;
int bytesPerPixel = 1;
int size = width * height * channels * bytesPerPixel;
unsigned char* data = new unsigned char[size];
if (!data) {
qCritical() << "Failed to allocate memory for raw data";
fclose(f);
return;
}
if (fread(data, 1, size, f) != size) {
qCritical() << "Failed to read raw data from file";
delete[] data;
fclose(f);
return;
}
fclose(f);
// Create texture
m_texture.reset(new QOpenGLTexture(QOpenGLTexture::Target2D));
m_texture->setSize(width, height);
m_texture->setFormat(QOpenGLTexture::R8_UNorm);
m_texture->setWrapMode(QOpenGLTexture::ClampToEdge);
m_texture->setMinificationFilter(QOpenGLTexture::Nearest);
m_texture->setMagnificationFilter(QOpenGLTexture::Nearest);
m_texture->allocateStorage();
m_texture->setData(QOpenGLTexture::Red, QOpenGLTexture::UInt8, data);
delete[] data;
// Create shader program
m_shaderProgram.reset(new QOpenGLShaderProgram());
m_shaderProgram->addShaderFromSourceCode(QOpenGLShader::Vertex,
"attribute vec3 a_position;\n"
"attribute vec2 a_texcoord;\n"
"varying vec2 v_texcoord;\n"
"void main() {\n"
" gl_Position = vec4(a_position, 1.0);\n"
" v_texcoord = a_texcoord;\n"
"}");
m_shaderProgram->addShaderFromSourceCode(QOpenGLShader::Fragment,
"uniform sampler2D u_texture;\n"
"varying vec2 v_texcoord;\n"
"void main() {\n"
" gl_FragColor = texture2D(u_texture, v_texcoord);\n"
"}");
if (!m_shaderProgram->link()) {
qCritical() << "Failed to link shader program:" << m_shaderProgram->log();
return;
}
// Set up vertex data
GLfloat positions[] = {
-1.0f, -1.0f, 0.0f,
1.0f, -1.0f, 0.0f,
-1.0f, 1.0f, 0.0f,
1.0f, 1.0f, 0.0f
};
GLfloat texcoords[] = {
0.0f, 1.0f,
1.0f, 1.0f,
0.0f, 0.0f,
1.0f, 0.0f
};
glGenBuffers(1, &m_vertexBuffer);
glBindBuffer(GL_ARRAY_BUFFER, m_vertexBuffer);
glBufferData(GL_ARRAY_BUFFER, sizeof(positions) + sizeof(texcoords), nullptr, GL_STATIC_DRAW);
glBufferSubData(GL_ARRAY_BUFFER, 0, sizeof(positions), positions);
glBufferSubData(GL_ARRAY_BUFFER, sizeof(positions), sizeof(texcoords), texcoords);
glBindBuffer(GL_ARRAY_BUFFER, 0);
// Set up vertex array object
glGenVertexArrays(1, &m_vertexArray);
glBindVertexArray(m_vertexArray);
glBindBuffer(GL_ARRAY_BUFFER, m_vertexBuffer);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, nullptr);
glEnableVertexAttribArray(0);
glVertexAttribPointer(1, 2, GL_FLOAT, GL_FALSE, 0, reinterpret_cast<void*>(sizeof(positions)));
glEnableVertexAttribArray(1);
glBindBuffer(GL_ARRAY_BUFFER, 0);
glBindVertexArray(0);
// Set clear color
glClearColor(0.0f, 0.0f, 0.0f, 0.0f);
}
void paintGL() override
{
glClear(GL_COLOR_BUFFER_BIT);
m_shaderProgram->bind();
m_texture->bind();
glBindVertexArray(m_vertexArray);
glDrawArrays(GL_TRIANGLE_STRIP, 0, 4);
glBindVertexArray(0);
m_texture->release();
m_shaderProgram->release();
}
private:
QString m_rawFilePath;
QScopedPointer<QOpenGLTexture> m_texture;
QScopedPointer<QOpenGLShaderProgram> m_shaderProgram;
GLuint m_vertexBuffer = 0;
GLuint m_vertexArray = 0;
};
int main(int argc, char* argv[])
{
QApplication app(argc, argv);
RawToRgbWidget widget("path/to/raw/file", nullptr);
widget.show();
return app.exec();
}
```
在上面的代码中,我们使用了QOpenGLWidget来创建OpenGL上下文,并在其中创建纹理和着色器程序,然后将纹理绑定到着色器程序中进行渲染。这个例子中的RAW文件是一个灰度图像,所以我们只需要使用单个通道纹理。如果你的RAW文件是带有彩色数据的,你需要使用三个通道的纹理来表示R、G、B三个通道的数据。
阅读全文
相关推荐
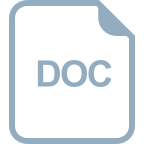
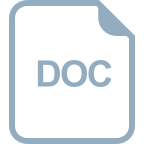
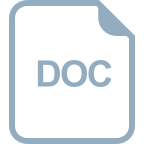
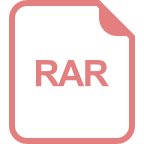
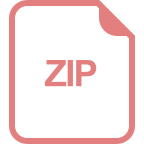
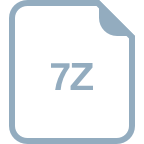
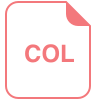
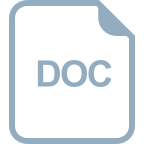
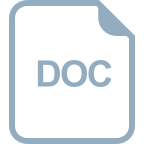
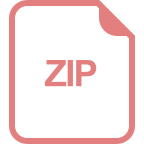
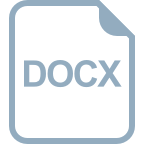
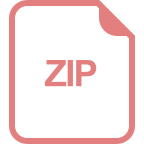
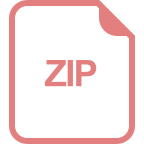
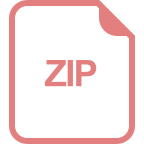