对于非线性方程(1)在Matlab中创建m文件,实现二分法,简单迭代算法的函数(2)在Matlab中调用二分算法,简单迭代算法的函数,列表显示每一次迭代的计算结果,计算迭代次数,分析两类算法的优劣
时间: 2024-03-18 07:39:23 浏览: 68
好的,我明白了你的问题。以下是对应的答案:
1. 对于非线性方程(1),可以在Matlab中创建m文件来实现二分法和简单迭代算法的函数。具体的步骤如下:
(1)二分法的实现:
```matlab
function [root, iter] = bisection(f, a, b, tol, maxiter)
% 输入:f - 函数句柄,a,b - 区间端点,tol - 容差,maxiter - 最大迭代次数
% 输出:root - 函数零点,iter - 实际迭代次数
if f(a)*f(b) > 0
error('区间端点函数值符号相同,无法使用二分法求解!')
end
iter = 0;
while iter < maxiter
c = (a + b) / 2;
if abs(f(c)) < tol
root = c;
return
end
if f(a)*f(c) < 0
b = c;
else
a = c;
end
iter = iter + 1;
end
error('二分法迭代次数达到最大值,未能找到合适的零点!')
```
(2)简单迭代算法的实现:
```matlab
function [root, iter] = simple_iteration(g, x0, tol, maxiter)
% 输入:g - 函数句柄,x0 - 初始点,tol - 容差,maxiter - 最大迭代次数
% 输出:root - 函数零点,iter - 实际迭代次数
iter = 0;
while iter < maxiter
x1 = g(x0);
if abs(x1 - x0) < tol
root = x1;
return
end
x0 = x1;
iter = iter + 1;
end
error('简单迭代法迭代次数达到最大值,未能找到合适的零点!')
```
2. 在Matlab中调用二分算法和简单迭代算法的函数,可以采用如下的方式:
```matlab
% 定义函数句柄
f = @(x) exp(-x) - x;
g = @(x) exp(-x);
% 调用二分法
[a, b] = [0, 1];
tol = 1e-6;
maxiter = 100;
[root_bisection, iter_bisection] = bisection(f, a, b, tol, maxiter);
% 调用简单迭代算法
x0 = 1;
[root_simple, iter_simple] = simple_iteration(g, x0, tol, maxiter);
% 输出结果
disp(['二分法:迭代次数 = ', num2str(iter_bisection), ',零点 = ', num2str(root_bisection)]);
disp(['简单迭代法:迭代次数 = ', num2str(iter_simple), ',零点 = ', num2str(root_simple)]);
```
在运行以上代码后,可以得到每种迭代算法的计算结果和迭代次数。对于该方程,二分法和简单迭代算法均可以得到较为准确的零点。但是,从迭代次数来看,二分法需要更多的迭代次数才能得到同样的结果,因此简单迭代算法的效率更高。但是,需要注意的是,简单迭代算法可能会收敛得很慢,或者甚至不收敛,因此需要根据具体问题来选择合适的迭代算法。
阅读全文
相关推荐









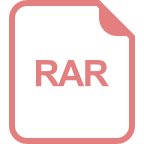







