void ADC_Activate(void) { __IO uint32_t wait_loop_index = 0U; #if (USE_TIMEOUT == 1) uint32_t Timeout = 0U; /* Variable used for timeout management / #endif / USE_TIMEOUT / /## Operation on ADC hierarchical scope: ADC instance #####################/ / Note: Hardware constraint (refer to description of the functions / / below): / / On this STM32 series, setting of these features is conditioned to / / ADC state: / / ADC must be disabled. / / Note: In this example, all these checks are not necessary but are / / implemented anyway to show the best practice usages / / corresponding to reference manual procedure. / / Software can be optimized by removing some of these checks, if / / they are not relevant considering previous settings and actions / / in user application. / if (LL_ADC_IsEnabled(ADC1) == 0) { / Run ADC self calibration / LL_ADC_StartCalibration(ADC1, LL_ADC_CALIB_OFFSET); / Poll for ADC effectively calibrated / #if (USE_TIMEOUT == 1) Timeout = ADC_CALIBRATION_TIMEOUT_MS; #endif / USE_TIMEOUT / while (LL_ADC_IsCalibrationOnGoing(ADC1) != 0) { #if (USE_TIMEOUT == 1) / Check Systick counter flag to decrement the time-out value / if (LL_SYSTICK_IsActiveCounterFlag()) { if(Timeout-- == 0) { / Error: Time-out / Error_Handler(); } } #endif / USE_TIMEOUT / } / Delay between ADC end of calibration and ADC enable. / / Note: Variable divided by 2 to compensate partially / / CPU processing cycles (depends on compilation optimization). / wait_loop_index = (ADC_DELAY_CALIB_ENABLE_CPU_CYCLES >> 1); while(wait_loop_index != 0) { wait_loop_index--; } / Enable ADC / LL_ADC_Enable(ADC1); / Poll for ADC ready to convert / #if (USE_TIMEOUT == 1) Timeout = ADC_ENABLE_TIMEOUT_MS; #endif / USE_TIMEOUT / while (LL_ADC_IsActiveFlag_ADRDY(ADC1) == 0) { #if (USE_TIMEOUT == 1) / Check Systick counter flag to decrement the time-out value / if (LL_SYSTICK_IsActiveCounterFlag()) { if(Timeout-- == 0) { / Error: Time-out / Error_Handler(); } } #endif / USE_TIMEOUT / } / Note: ADC flag ADRDY is not cleared here to be able to check ADC / / status afterwards. / / This flag should be cleared at ADC Deactivation, before a new / / ADC activation, using function "LL_ADC_ClearFlag_ADRDY()". */ }请逐行解释代码
时间: 2024-03-16 17:43:52 浏览: 14
这是一段STM32的C语言代码,用于激活ADC(模数转换器)并进行自校准。下面对每行代码进行逐行解释:
```c
void ADC_Activate(void) {
```
这是一个函数的定义,函数名为ADC_Activate,不带参数,返回类型为void(即无返回值)。
```c
__IO uint32_t wait_loop_index = 0U;
```
定义一个无符号32位整数类型的变量wait_loop_index,并初始化为0。
```c
#if (USE_TIMEOUT == 1)
uint32_t Timeout = 0U;
```
如果USE_TIMEOUT宏定义为1,则定义一个无符号32位整数类型的变量Timeout,并初始化为0。
```c
#endif /* USE_TIMEOUT */
```
结束USE_TIMEOUT的条件编译。
```c
if (LL_ADC_IsEnabled(ADC1) == 0) {
```
如果ADC1没有被启用,则执行以下代码。
```c
LL_ADC_StartCalibration(ADC1, LL_ADC_CALIB_OFFSET);
```
启动ADC1的自校准,使用偏移校准模式。
```c
#if (USE_TIMEOUT == 1)
Timeout = ADC_CALIBRATION_TIMEOUT_MS;
#endif /* USE_TIMEOUT */
```
如果USE_TIMEOUT宏定义为1,则将Timeout变量设置为ADC自校准的超时时间。
```c
while (LL_ADC_IsCalibrationOnGoing(ADC1) != 0) {
```
等待ADC1的自校准完成。
```c
#if (USE_TIMEOUT == 1)
if (LL_SYSTICK_IsActiveCounterFlag()) {
if(Timeout-- == 0) {
Error_Handler();
}
}
#endif /* USE_TIMEOUT */
```
如果USE_TIMEOUT宏定义为1,则检查系统计时器的计数标志位,如果超时则调用Error_Handler函数。
```c
wait_loop_index = (ADC_DELAY_CALIB_ENABLE_CPU_CYCLES >> 1);
while(wait_loop_index != 0) {
wait_loop_index--;
}
```
为了减少CPU处理周期的影响,等待一段时间再启用ADC1。ADC_DELAY_CALIB_ENABLE_CPU_CYCLES是一个宏定义,表示需要等待的CPU周期数。
```c
LL_ADC_Enable(ADC1);
```
启用ADC1。
```c
#if (USE_TIMEOUT == 1)
Timeout = ADC_ENABLE_TIMEOUT_MS;
#endif /* USE_TIMEOUT */
```
如果USE_TIMEOUT宏定义为1,则将Timeout变量设置为ADC启用的超时时间。
```c
while (LL_ADC_IsActiveFlag_ADRDY(ADC1) == 0) {
```
等待ADC1准备好进行转换。
```c
#if (USE_TIMEOUT == 1)
if (LL_SYSTICK_IsActiveCounterFlag()) {
if(Timeout-- == 0) {
Error_Handler();
}
}
#endif /* USE_TIMEOUT */
```
如果USE_TIMEOUT宏定义为1,则检查系统计时器的计数标志位,如果超时则调用Error_Handler函数。
```c
}
```
函数结束。
相关推荐
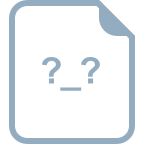
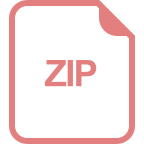
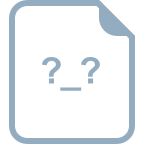














