用kivy写一个手机记账app
时间: 2023-12-10 07:03:16 浏览: 62
好的,下面是一个简单的用Kivy写的手机记账App的代码示例。这个App允许用户输入账单明细、日期和费用,并将其保存到本地文件中。
```python
import os
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.properties import ObjectProperty
from kivy.utils import get_color_from_hex
from kivy.core.window import Window
class AddExpenseForm(BoxLayout):
expense_title = ObjectProperty()
expense_date = ObjectProperty()
expense_amount = ObjectProperty()
def __init__(self, **kwargs):
super(AddExpenseForm, self).__init__(**kwargs)
def validate_input(self):
if not self.expense_title.text:
self.expense_title.hint_text = "Title is required"
self.expense_title.hint_text_color = get_color_from_hex('#FF0000')
return False
elif not self.expense_date.text:
self.expense_date.hint_text = "Date is required"
self.expense_date.hint_text_color = get_color_from_hex('#FF0000')
return False
elif not self.expense_amount.text:
self.expense_amount.hint_text = "Amount is required"
self.expense_amount.hint_text_color = get_color_from_hex('#FF0000')
return False
else:
return True
def save_expense(self):
if self.validate_input():
expense_data = self.expense_title.text + ',' + self.expense_date.text + ',' + self.expense_amount.text
with open("expenses.txt", "a") as f:
f.write(expense_data + "\n")
self.expense_title.text = ""
self.expense_date.text = ""
self.expense_amount.text = ""
class ExpenseManager(App):
def build(self):
Window.clearcolor = get_color_from_hex('#FFFFFF')
if not os.path.exists("expenses.txt"):
open("expenses.txt", "w").close()
return AddExpenseForm()
if __name__ == '__main__':
ExpenseManager().run()
```
在这个App中,我们使用了一个BoxLayout来创建一个简单的表单,用于输入账单明细、日期和费用。当用户点击“Save”按钮时,我们会验证用户输入是否有效,并将账单数据保存到本地文件中。
运行这个App,你将看到一个简单的表单,输入账单信息并点击“Save”按钮即可保存。
阅读全文
相关推荐
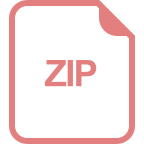
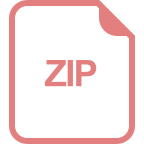
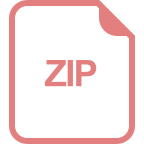















