Write the following code using c #, Add another method called ShowMenu() to your project.This method will call the method in question 7 continuously until the user presses 0. (You will have to invoke the method in a loop body, read in the user input as well as check the input). You will need to hook up all the methods that you wrote previously i.e. questions 1 to 6.Replace all the code from your Main() method with a single call to the ShowMenu() method.
时间: 2024-02-13 22:01:46 浏览: 87
Sure, here's an example implementation in C#:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
ShowMenu();
}
static void ShowMenu()
{
int choice;
do
{
Console.WriteLine("1. Example method 1");
Console.WriteLine("2. Example method 2");
Console.WriteLine("3. Example method 3");
Console.WriteLine("4. Example method 4");
Console.WriteLine("5. Example method 5");
Console.WriteLine("6. Example method 6");
Console.WriteLine("0. Exit");
Console.Write("Enter choice: ");
if (int.TryParse(Console.ReadLine(), out choice))
{
switch (choice)
{
case 1:
// Call method 1
break;
case 2:
// Call method 2
break;
case 3:
// Call method 3
break;
case 4:
// Call method 4
break;
case 5:
// Call method 5
break;
case 6:
// Call method 6
break;
case 0:
Console.WriteLine("Exiting program...");
break;
default:
Console.WriteLine("Invalid choice.");
break;
}
}
else
{
Console.WriteLine("Invalid input. Please enter a number.");
}
Console.WriteLine();
} while (choice != 0);
}
}
```
Note that you will need to replace the comments in the switch statement with the actual method calls for your specific project.
阅读全文
相关推荐
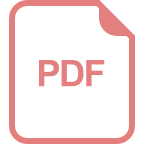
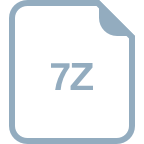
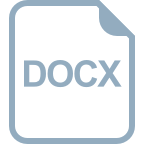
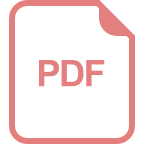
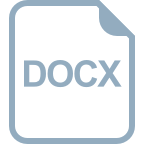
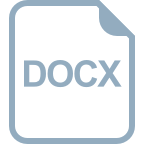
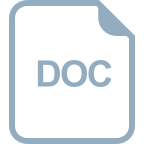












