用java代码写一个多线程下载文件接口
时间: 2023-05-16 21:05:15 浏览: 63
好的,以下是一个简单的多线程下载文件接口的 Java 代码:
```java
import java.io.*;
import java.net.URL;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class MultiThreadedDownloader {
private static final int BUFFER_SIZE = 4096;
private static final int NUM_THREADS = 4;
public static void download(String fileUrl, String saveDir) throws IOException {
URL url = new URL(fileUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
int responseCode = conn.getResponseCode();
if (responseCode != HttpURLConnection.HTTP_OK) {
throw new IOException("Server returned HTTP response code: " + responseCode);
}
String fileName = getFileName(conn);
String filePath = saveDir + File.separator + fileName;
ExecutorService executor = Executors.newFixedThreadPool(NUM_THREADS);
int fileSize = conn.getContentLength();
int numParts = fileSize / BUFFER_SIZE + 1;
for (int i = 0; i < numParts; i++) {
int start = i * BUFFER_SIZE;
int end = Math.min((i + 1) * BUFFER_SIZE, fileSize);
executor.execute(new DownloadTask(url, filePath, start, end));
}
executor.shutdown();
while (!executor.isTerminated()) {
}
System.out.println("File downloaded to: " + filePath);
}
private static String getFileName(HttpURLConnection conn) {
String fileName = "";
String disposition = conn.getHeaderField("Content-Disposition");
String contentType = conn.getContentType();
int contentLength = conn.getContentLength();
if (disposition != null) {
int index = disposition.indexOf("filename=");
if (index > 0) {
fileName = disposition.substring(index + 10, disposition.length() - 1);
}
} else {
fileName = conn.getURL().getFile();
fileName = fileName.substring(fileName.lastIndexOf("/") + 1);
}
return fileName;
}
private static class DownloadTask implements Runnable {
private URL url;
private String filePath;
private int start;
private int end;
public DownloadTask(URL url, String filePath, int start, int end) {
this.url = url;
this.filePath = filePath;
this.start = start;
this.end = end;
}
@Override
public void run() {
try {
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Range", "bytes=" + start + "-" + end);
InputStream inputStream = conn.getInputStream();
RandomAccessFile file = new RandomAccessFile(filePath, "rw");
file.seek(start);
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
file.write(buffer, 0, bytesRead);
}
file.close();
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
你可以调用 `MultiThreadedDownloader.download(fileUrl, saveDir)` 方法来下载文件,其中 `fileUrl` 是文件的 URL,`saveDir` 是保存文件的目录。这个方法会启动多个线程来下载文件,提高下载速度。
相关推荐
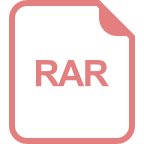
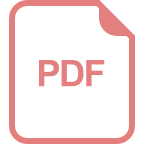
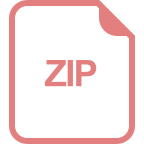

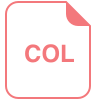
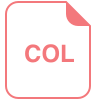
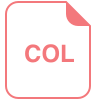
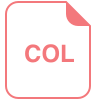









