线性表基本操作的源代码
时间: 2023-05-25 08:03:51 浏览: 58
以下是线性表的基本操作的源代码:
//定义线性表结构体
typedef struct LinearList{
int *data; //指向动态数组的指针
int size; //线性表当前长度
int capacity; //线性表最大长度
}List;
//初始化线性表
void initList(List *list, int capacity){
list -> data = (int *)malloc(capacity * sizeof(int));
list -> size = 0;
list -> capacity = capacity;
}
//判断线性表是否为空
bool isEmpty(List *list){
return list -> size == 0;
}
//判断线性表是否已满
bool isFull(List *list){
return list -> size == list -> capacity;
}
//获取线性表当前长度
int getListSize(List *list){
return list -> size;
}
//获取线性表最大长度
int getListCapacity(List *list){
return list -> capacity;
}
//获取指定位置的元素
int getElement(List *list, int index){
if(index >= 0 && index < list -> size){
return list -> data[index];
}else{
return INT_MIN; //返回(int类型)最小值,表示非法输入
}
}
//插入元素
bool insert(List *list, int index, int element){
if(isFull(list)){
return false;
}
if(index >= 0 && index <= list -> size){
for(int i = list -> size - 1; i >= index; i--){
list -> data[i + 1] = list -> data[i];
}
list -> data[index] = element;
list -> size++;
return true;
}else{
return false;
}
}
//删除指定位置的元素
bool deleteElement(List *list, int index){
if(isEmpty(list)){
return false;
}
if(index >= 0 && index < list -> size){
for(int i = index; i < list -> size - 1; i++){
list -> data[i] = list -> data[i + 1];
}
list -> size--;
return true;
}else{
return false;
}
}
//销毁线性表,释放动态数组内存
void destroyList(List *list){
free(list -> data);
list -> size = 0;
list -> capacity = 0;
}
相关推荐
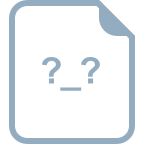














