基于深度学习YOLOv3完成车牌检测识别出的车牌用方框圈出来 代码
时间: 2023-09-20 07:12:45 浏览: 215
以下是基于YOLOv3完成车牌检测并用方框圈出的示例代码:
```python
import cv2
import numpy as np
# 载入预训练权重
net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
# 加载 COCO 数据集标签
classes = []
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 车牌的类别
plate_class = 'license plate'
# 加载测试图像
img = cv2.imread('test.jpg')
# 获取图像尺寸
height, width, _ = img.shape
# 构建输入图像的blob
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
# 设置网络的输入
net.setInput(blob)
# 运行前向传递,获取网络的输出
outs = net.forward(net.getUnconnectedOutLayersNames())
# 遍历所有检测结果
for out in outs:
# 遍历每个检测结果
for detection in out:
# 获取类别的置信度
scores = detection[5:]
# 获取最大置信度的类别
class_id = np.argmax(scores)
# 如果检测到的类别是车牌,进行车牌检测
if classes[class_id] == plate_class:
# 获取检测结果的坐标
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
# 计算轮廓框的左上角坐标
x = int(center_x - w/2)
y = int(center_y - h/2)
# 绘制方框
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示结果
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:以上代码仅为示例,具体实现需要根据实际情况进行调整。
阅读全文
相关推荐
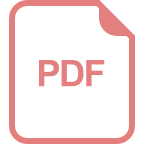
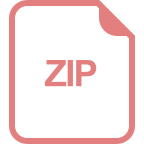
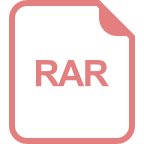
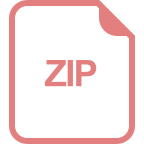
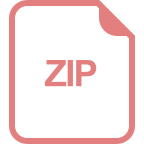
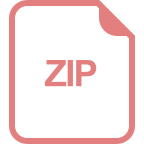
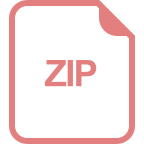
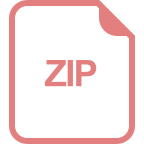
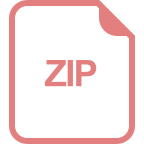
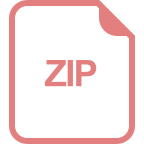
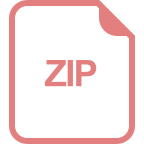
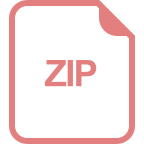
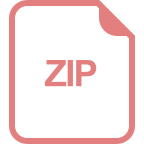
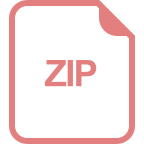
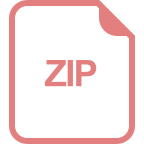
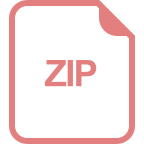