ü定义一个基类person,保护数据成员:name(姓名),sex(性别),age(年龄),构造函数。 ü派生出教师Teacher类,保护数据成员:title(职称),构造函数。 ü派生出Student类,保护数据成员:score(成绩),构造函数。 ü用多继承的方式定义一个在职研究生(Graduate)派生类私有数据成员wage(工资),构造函数和公有成员函数show()。
时间: 2024-01-09 17:04:45 浏览: 89
好的,这是一个面向对象的编程问题,我会尽力回答。
以下是C++的代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 基类 Person
class Person {
protected:
string name;
string sex;
int age;
public:
Person(string name, string sex, int age) {
this->name = name;
this->sex = sex;
this->age = age;
}
};
// 教师类 Teacher
class Teacher : public Person {
protected:
string title;
public:
Teacher(string name, string sex, int age, string title) : Person(name, sex, age) {
this->title = title;
}
};
// 学生类 Student
class Student : public Person {
protected:
int score;
public:
Student(string name, string sex, int age, int score) : Person(name, sex, age) {
this->score = score;
}
};
// 在职研究生类 Graduate,多继承 Person 和 Teacher 类,私有继承
class Graduate : private Person, private Teacher {
private:
int wage;
public:
Graduate(string name, string sex, int age, string title, int wage) : Person(name, sex, age), Teacher(name, sex, age, title) {
this->wage = wage;
}
void show() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "年龄:" << age << endl;
cout << "职称:" << title << endl;
cout << "工资:" << wage << endl;
}
};
```
在这个代码中,我们定义了一个基类 Person,它有三个保护数据成员:name、sex、age,并且有一个构造函数。然后我们派生出了教师类 Teacher,它有一个保护数据成员 title,并且也有一个构造函数。接着,我们又派生出了学生类 Student,它有一个保护数据成员 score,并且也有一个构造函数。
最后,我们用多继承的方式定义了一个在职研究生类 Graduate。它继承了 Person 和 Teacher 两个类,并且私有继承。它还定义了一个私有数据成员 wage,并且有一个构造函数。在这个类中,我们还定义了一个公有成员函数 show(),用于输出这个在职研究生的信息。
需要注意的是,由于 Graduate 类私有继承了 Person 和 Teacher 两个类,因此在 Graduate 类中,我们不能直接访问 Person 和 Teacher 类中的保护数据成员和成员函数。我们只能通过 Graduate 类的构造函数来初始化这些数据成员。但是,在 Graduate 类中,我们可以访问 Person 和 Teacher 类中的公有成员函数。
阅读全文
相关推荐
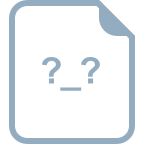
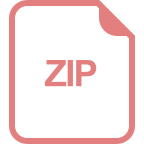
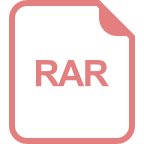
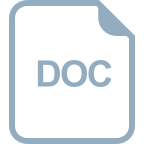
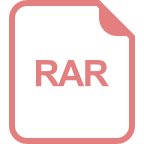
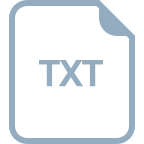
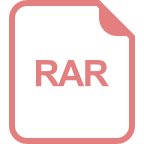
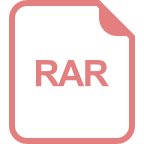
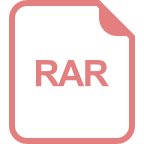
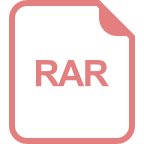
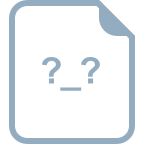
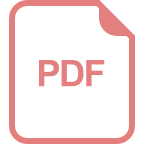
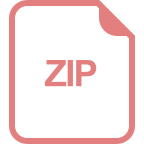
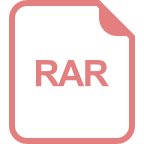
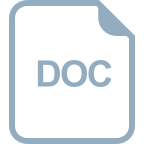
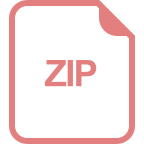