mapreduce实现wordcount程序
时间: 2023-04-24 19:05:33 浏览: 251
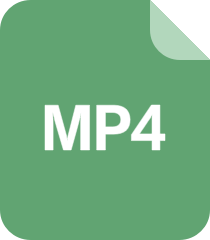
03.mapreduce实现wordcount的逻辑代码.mp4
MapReduce是一种分布式计算模型,可以用来处理大规模数据集。WordCount程序是MapReduce的经典案例之一,用于统计文本中每个单词出现的次数。
实现WordCount程序的步骤如下:
1. Map阶段:将输入的文本分割成单词,对每个单词进行标记,输出键值对<单词,1>。
2. Shuffle阶段:将Map阶段输出的键值对按照键进行排序,将相同键的值合并成一个列表。
3. Reduce阶段:对每个键值对的值列表进行求和,输出键值对<单词,出现次数>。
通过以上三个步骤,就可以实现WordCount程序。在Hadoop中,可以使用Java或者其他编程语言来实现MapReduce程序。
阅读全文
相关推荐
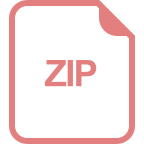
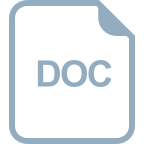
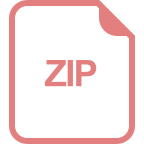
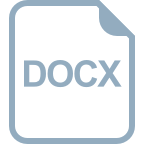
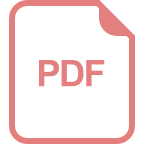
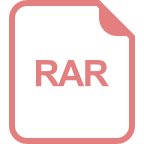
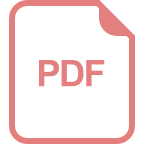
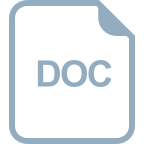







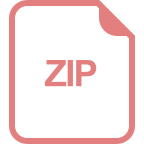