private BluetoothServer.OnAcceptListener onAcceptListener = new BluetoothServer.OnAcceptListener() { @SuppressLint("MissingPermission") @Override public void onAccepted(BluetoothSocket socket) { btManager = new BluetoothManager(socket); btManager.setServer(true); btManager.setOnReceivedListener(onReceivedListener); btManager.setOnEventListener(onEventListener); Toast.makeText(MainActivity.this,"收到一个蓝牙连接!",Toast.LENGTH_LONG).show(); setTitle("蓝牙连接到:" + btManager.getRemoteName()); if(bluetoothAddress == null){ BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); bluetoothAddress = bluetoothAdapter.getAddress(); } refreshData(); } };
时间: 2024-02-14 15:20:26 浏览: 85
这段代码是关于蓝牙连接的,具体来说是在Android的MainActivity中创建了一个蓝牙服务器,并设置了一个OnAcceptListener监听器来监听是否有客户端连接。当有客户端连接时,会创建一个BluetoothManager对象,设置一些监听器,并显示一个Toast提示收到了蓝牙连接。同时,会将蓝牙连接的远程名称设置为MainActivity的标题,并更新数据。请问这段代码中的BluetoothManager是什么?
相关问题
// 初始化service // gatt 回调 GattCallback.Refresh f = new GattCallback.Refresh() { private static final String TAG = "GattCallback Gatt"; @Override public void OperationCv(BluetoothGattCharacteristic cvRead, BluetoothGattCharacteristic cvWrite, BluetoothGattCharacteristic cvConfig) { } @Override public void Operation(boolean isRead, boolean isWrite, boolean isConfig) { // 更新操作界面 showOpView(isRead, isWrite); }解释代码
这段代码是一个Bluetooth GATT(Generic Attribute Profile)回调的实现示例。
首先,它包含一个GattCallback.Refresh接口实现,其中Refresh是自定义的接口名。在此代码中,该接口包含两个方法:
1. OperationCv(BluetoothGattCharacteristic cvRead, BluetoothGattCharacteristic cvWrite, BluetoothGattCharacteristic cvConfig)
其中,cvRead、cvWrite和cvConfig都是BluetoothGattCharacteristic类型的参数。这个方法看起来是空的,没有实现任何功能,但可能在某些情况下需要覆盖它。
2. Operation(boolean isRead, boolean isWrite, boolean isConfig)
这个方法包含三个布尔类型的参数:isRead、isWrite和isConfig。这些参数表示是否支持读、写和配置操作。然后,这个方法会调用showOpView(isRead, isWrite)方法来更新操作界面。
总体而言,这段代码是在初始化Bluetooth GATT服务,并实现了一个自定义的GattCallback.Refresh接口,以便在进行读写和配置操作时更新操作界面。
安卓开发之bluetooth
### Android 开发中的蓝牙功能概述
在 Android 平台下,蓝牙技术主要用于实现设备间的短距离无线通信。对于开发者而言,掌握如何利用蓝牙进行数据传输至关重要。
#### 蓝牙适配器初始化
为了操作蓝牙硬件,应用程序必须先获取 `BluetoothAdapter` 实例来管理蓝牙连接和其他相关配置:
```java
// 获取默认的蓝牙适配器实例
final BluetoothManager bluetoothManager =
(BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter mBluetoothAdapter = bluetoothManager.getAdapter();
```
当尝试访问蓝牙特性前,应当验证是否存在可用的蓝牙模块以及其状态是否正常工作[^3]。
#### 启用蓝牙服务
确保蓝牙处于启用状态是建立任何蓝牙会话的前提条件之一。通过调用 `isEnabled()` 方法可判断当前蓝牙开关的状态;若返回 false,则表示蓝牙尚未激活,此时可以通过发送意图请求用户手动打开它:
```java
if (!mBluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
```
这段代码片段展示了怎样引导用户进入系统的蓝牙设置界面并允许他们启动蓝牙支持。
#### 构建 GATT 服务器端应用
针对低功耗蓝牙(BLE),构建一个通用属性协议(GATT)服务器可以让其他客户端与其交互和服务发现过程更加高效。具体来说,在 Android Things 上创建这样的服务器涉及定义特征值、描述符及其对应的权限级别等内容,并最终注册到本地数据库中等待远程设备发起查询或写入命令[^1]。
例如,下面是一个简单的 Java 类用于演示如何搭建基本结构:
```java
public class MyGattServer extends Application {
private static final String SERVICE_UUID = "0000fff0-0000-1000-8000-00805f9b34fb";
@Override
public void onCreate() {
super.onCreate();
BluetoothLeAdvertiser advertiser = BluetoothAdapter.getDefaultAdapter().getBluetoothLeAdvertiser();
// 设置广告参数...
BluetoothGattService service = new BluetoothGattService(UUID.fromString(SERVICE_UUID),
BluetoothGattService.SERVICE_TYPE_PRIMARY);
// 添加更多特征...
BluetoothGatt gattServer = BluetoothManager.getInstance()
.openGattServer(this, new BluetoothGattServerCallback());
gattServer.addService(service);
// 开始广播...
}
}
```
此段代码仅作为概念证明展示了一些必要的组件声明方式,实际项目可能还需要考虑更多的细节处理逻辑。
#### 使用 RFCOMM 协议
除了 BLE 外,传统蓝牙也提供了多种不同的通讯模式供选择,其中最常见的是串行端口仿真——即 RFCOMM。这种基于 TCP/IP 的流套接字机制非常适合于那些需要稳定可靠的数据交换场景下的应用开发。有关 RFCOMM 更多的技术规格说明可以在官方文档找到[^2]。
阅读全文
相关推荐









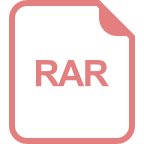






