Python字符串中去除空格
时间: 2023-08-27 09:33:42 浏览: 90
可以使用 `replace()` 方法将字符串中的空格替换成空字符串:
```python
text = " Hello World! "
clean_text = text.replace(" ", "")
print(clean_text)
```
输出结果为:
```
HelloWorld!
```
这里将空格替换成空字符串,实现了去除空格的效果。如果你想只去除字符串两端的空格,可以使用 `strip()` 方法:
```python
text = " Hello World! "
clean_text = text.strip()
print(clean_text)
```
输出结果为:
```
Hello World!
```
这里使用了 `strip()` 方法,它会去除字符串两端的空格,但不会去除字符串中间的空格。
相关问题
Python字符串去除前后空格
在Python中,你可以使用内置的`strip()`函数来去除字符串前后多余的空格。这个方法会移除字符串开头和结尾的所有空白字符,包括空格、制表符和换行符。例如:
```python
s = " hello world "
stripped_s = s.strip()
print(stripped_s) # 输出: "hello world"
```
如果你想移除所有连续的空格,可以使用`replace()`函数替换为空或者使用正则表达式`re`模块:
```python
import re
s = " a b c d e f g "
no_extra_spaces = ' '.join(s.split())
or_with_regex = re.sub(r'\s+', ' ', s)
print(no_extra_spaces) # 输出: "a b c d e f g"
print(or_with_regex) # 输出: "a b c d e f g"
```
python字符串中如何去除空格
可以使用字符串的 `strip()` 方法去除字符串中的空格,该方法会去除字符串开头和结尾的空格。例如:
```python
s = " hello world "
s = s.strip()
print(s) # 输出:hello world
```
如果要去除字符串中间的空格,可以使用字符串的 `replace()` 方法,将空格替换为空字符串即可。例如:
```python
s = "hello world"
s = s.replace(" ", "")
print(s) # 输出:helloworld
```
另外,如果要去除特定位置的空格,可以使用字符串的切片操作,将需要去除的空格从字符串中切掉。例如:
```python
s = "hello world"
s = s[:5] + s[10:]
print(s) # 输出:hello world
```
阅读全文
相关推荐
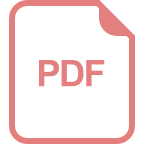
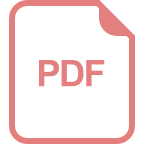
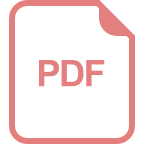
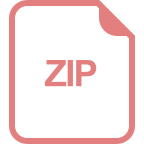
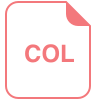
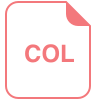










