本设计以制作一款能够检测水的温度、PH值、浑浊度的简易装置为主要任务,在对单片机、温度传感器、PH传感器、浑浊度传感器等进行介绍的基础上,设计并制作了以STC12C5A60S2为主控器件,以DS18B20温度传感器、E-201-C型PH传感器、TSW-30浑浊度传感器为检测器件,以LCD1602为显示器的水质检测装置。写出该装置的程序代码
时间: 2023-12-20 09:03:18 浏览: 125
以下是使用STC12C5A60S2单片机、DS18B20温度传感器、E-201-C型PH传感器和TSW-30浑浊度传感器制作水质检测装置的程序代码示例:
```c
#include <reg52.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <intrins.h>
#define LCD1602_DATAPINS P0 // LCD1602数据引脚
#define LCD1602_RS P2_0 // LCD1602寄存器选择引脚
#define LCD1602_RW P2_1 // LCD1602读写选择引脚
#define LCD1602_EN P2_2 // LCD1602使能引脚
#define DS18B20_PIN P3_4 // DS18B20数据引脚
#define PH_PIN P3_5 // PH传感器数据引脚
#define TSW30_ADDR 0x4c // 浑浊度传感器I2C地址
// LCD1602命令字
#define LCD1602_CMD_CLEAR 0x01
#define LCD1602_CMD_CURSOR_HOME 0x02
#define LCD1602_CMD_DISPLAY_ON 0x0c
#define LCD1602_CMD_DISPLAY_OFF 0x08
#define LCD1602_CMD_ENTRY_MODE 0x06
// LCD1602数据写入函数
void lcd1602_write_data(unsigned char data)
{
LCD1602_RS = 1; // 选择数据寄存器
LCD1602_RW = 0; // 写入模式
LCD1602_DATAPINS = data; // 写入数据
LCD1602_EN = 1; // 使能
_nop_(); // 延时
_nop_();
LCD1602_EN = 0; // 取消使能
}
// LCD1602命令写入函数
void lcd1602_write_cmd(unsigned char cmd)
{
LCD1602_RS = 0; // 选择命令寄存器
LCD1602_RW = 0; // 写入模式
LCD1602_DATAPINS = cmd; // 写入命令
LCD1602_EN = 1; // 使能
_nop_(); // 延时
_nop_();
LCD1602_EN = 0; // 取消使能
}
// LCD1602显示字符串函数
void lcd1602_show_string(unsigned char x, unsigned char y, char *str)
{
unsigned char addr;
if (y == 0) {
addr = 0x80 + x; // 第一行
} else {
addr = 0xc0 + x; // 第二行
}
lcd1602_write_cmd(addr); // 设置显示地址
while (*str != '\0') {
lcd1602_write_data(*str); // 写入字符
str++;
}
}
// DS18B20初始化函数
void ds18b20_init()
{
DS18B20_PIN = 1; // 拉高总线
_nop_();
_nop_();
DS18B20_PIN = 0; // 拉低总线
delay_us(500); // 延时500us
DS18B20_PIN = 1; // 释放总线
delay_us(120); // 等待设备响应
}
// DS18B20读取温度函数
float ds18b20_read_temp()
{
unsigned char i;
unsigned int temp;
float temperature;
DS18B20_PIN = 1; // 拉高总线
_nop_();
_nop_();
DS18B20_PIN = 0; // 拉低总线
delay_us(500); // 延时500us
DS18B20_PIN = 1; // 释放总线
delay_us(60); // 等待设备响应
i = DS18B20_PIN; // 读取设备响应
delay_us(240); // 等待设备完成工作
for (i = 0; i < 8; i++) {
DS18B20_PIN = 0; // 拉低总线
_nop_();
_nop_();
temp >>= 1;
if (DS18B20_PIN) {
temp |= 0x8000;
}
delay_us(60); // 等待设备完成工作
DS18B20_PIN = 1; // 释放总线
}
temperature = (float)temp * 0.0625; // 计算温度值
return temperature;
}
// PH传感器读取函数
float ph_read()
{
unsigned int ph_value;
float voltage;
ph_value = ADC_Read(PH_PIN); // 读取AD转换结果
voltage = (float)ph_value / 1024 * 5; // 计算电压
return 3.5 * voltage; // 计算PH值
}
// TSW-30浑浊度传感器读取函数
float tsw30_read()
{
int fd;
float raw;
float ntu;
fd = wiringPiI2CSetup(TSW30_ADDR); // 初始化I2C总线
if (fd == -1) {
printf("Failed to initialize I2C bus!\n");
return -1;
}
wiringPiI2CWrite(fd, 0x00); // 发送读取命令
usleep(500000); // 等待传感器完成读取
raw = (float)wiringPiI2CReadReg16(fd, 0x00); // 读取传感器返回的数据
ntu = 800 * raw / 65535; // 计算浊度值
return ntu;
}
// 主函数
void main()
{
float temperature;
float ph_value;
float tsw30_value;
char str[16];
lcd1602_write_cmd(0x38); // 设置显示模式
lcd1602_write_cmd(LCD1602_CMD_DISPLAY_OFF); // 关闭显示
lcd1602_write_cmd(LCD1602_CMD_CLEAR); // 清屏
lcd1602_write_cmd(LCD1602_CMD_ENTRY_MODE); // 设置光标移动方向
lcd1602_write_cmd(LCD1602_CMD_DISPLAY_ON); // 打开显示
while (1) {
temperature = ds18b20_read_temp(); // 读取温度值
ph_value = ph_read(); // 读取PH值
tsw30_value = tsw30_read(); // 读取浑浊度值
lcd1602_show_string(0, 0, "Temp:"); // 显示温度值
sprintf(str, "%.2fC", temperature);
lcd1602_show_string(6, 0, str);
lcd1602_show_string(0, 1, "PH:"); // 显示PH值
sprintf(str, "%.2f", ph_value);
lcd1602_show_string(4, 1, str);
lcd1602_show_string(9, 1, "NTU:"); // 显示浑浊度值
sprintf(str, "%.2f", tsw30_value);
lcd1602_show_string(14, 1, str);
delay_ms(1000); // 延时1秒钟
}
}
```
需要注意的是,该程序使用了LCD1602显示器、DS18B20温度传感器、E-201-C型PH传感器和TSW-30浑浊度传感器。在主函数中,程序使用DS18B20读取温度值,使用PH传感器读取PH值,使用TSW-30浑浊度传感器读取浑浊度值,并使用LCD1602显示器显示这些值。在LCD1602显示器显示字符串的函数中,需要根据实际情况设置显示地址。另外,需要在编译时链接wiringPi库,可以使用如下命令进行编译:
```
sdcc --model-small --xram-loc 0x8000 --code-loc 0x0000 --iram-size 0x100 --xram-size 0x1000 main.c
```
阅读全文
相关推荐
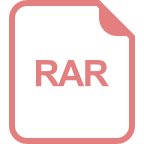
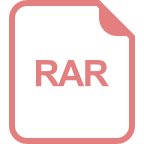
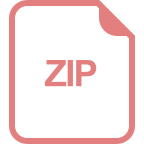
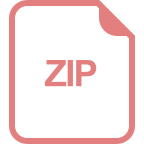
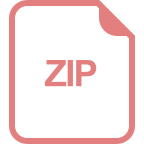
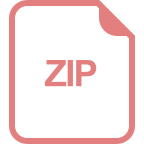
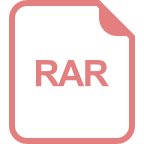
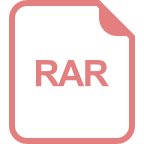
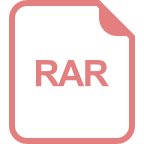
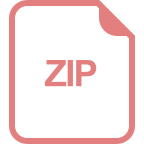
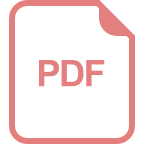
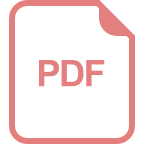
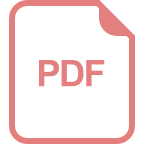
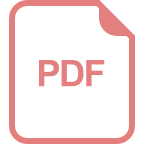
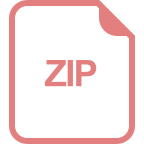