Fortran中使用泰勒级数求Fortran
时间: 2024-02-29 13:53:07 浏览: 91
好的,下面是一个使用泰勒级数求正弦函数的Fortran程序示例:
```fortran
program taylor_sin
implicit none
integer :: n, i
real :: x, sum, term
! 输入自变量x和级数项个数n
write(*, *) "请输入自变量x:"
read(*, *) x
write(*, *) "请输入级数项个数n:"
read(*, *) n
! 计算泰勒级数
sum = 0.0
term = x
do i = 1, n
sum = sum + term
term = -term * x * x / (2.0 * i) / (2.0 * i + 1.0)
end do
! 输出结果
write(*, *) "sin(", x, ") 的近似值为:", sum
end program taylor_sin
```
在这个示例中,我们使用了Fortran语言中的do循环来计算级数项的累加和,并且使用了一个额外的变量term来表示每个级数项的值。其中,我们使用了公式:$sin(x)=\sum_{n=0}^{\infty} \frac{(-1)^n x^{2n+1}}{(2n+1)!}$。你可以根据自己的需要修改自变量和级数项个数,以得到不同的近似值。
希望这个示例对你有所帮助!
相关问题
fortran泰勒级数
Fortran是一种编程语言,可以用它来实现泰勒级数的计算。泰勒级数是一种数学公式,它可以用来近似计算复杂的函数。Fortran提供了许多数学函数库和数值计算工具,可以方便地计算泰勒级数。下面是一个Fortran程序示例,用于计算sin(x)的泰勒级数:
```
program taylor_sin
implicit none
integer :: i, n
real :: x, term, sum
write(*,*) 'Enter the value of x:'
read(*,*) x
write(*,*) 'Enter the number of terms n:'
read(*,*) n
sum = 0.0
term = x
do i = 1, n
sum = sum + term
term = -term * x * x / ((2*i) * (2*i + 1))
end do
write(*,*) 'The value of sin(', x, ') is ', sum
end program taylor_sin
```
这个程序首先读取用户输入的x和n值,然后使用循环计算sin(x)的泰勒级数,并输出结果。在Fortran中,可以使用do循环来实现循环计算,也可以使用if语句等控制语句来实现流程控制。
fortran程序泰勒展开程序怎么写
Fortran是一种主要用于数值计算的编程语言,编写泰勒级数展开的程序通常涉及数学公式和循环结构。以下是一个简单的例子,展示如何使用Fortran编写一个函数,用于计算给定阶数的泰勒展开:
```fortran
module TaylorExpansion
implicit none
contains
subroutine TaylorPolynomial(n, x, f, dfdx)
integer, intent(in) :: n ! 展开的阶数
real(kind=8), intent(in) :: x ! 展开点
real(kind=8), intent(out) :: f ! 泰勒多项式的值
real(kind=8), dimension(:), intent(in) :: dfdx ! f(x+k*dx)的导数组成的向量,k = 0..n-1
real(kind=8) :: term, fact ! 单项式系数和阶乘因子
integer :: k
fact = 1.0_dp
f = 0.0_dp
do k = 0, n-1
term = dfdx(k) * (x**k) / fact
f = f + term
fact = fact * (k+1)
end do
end subroutine TaylorPolynomial
! 示例:假设dfdx存储了f(x+k*dx)的导数,这里我们仅给出一个基本的调用示例
real(kind=8) :: derivatives(5) ! 假设我们有一个5阶导数的数组
call TaylorPolynomial(4, 1.0_dp, 0.0_dp, derivatives)
!
阅读全文
相关推荐
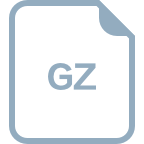
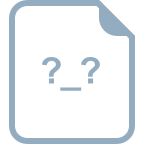
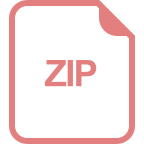
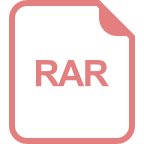
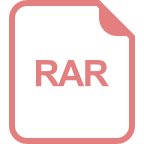
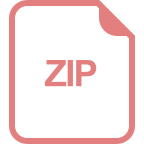
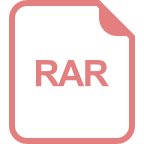
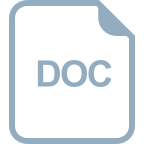
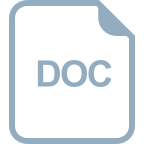
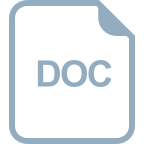
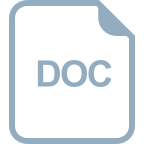
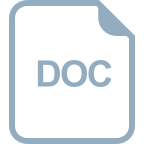
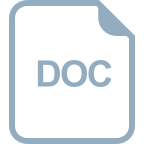
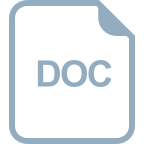
