java complete Read a .csv file
时间: 2024-05-08 20:16:55 浏览: 101
To read a .csv file in Java, you can use the following steps:
1. Create a new FileReader object to read the file, passing the file name or path as a string parameter.
2. Create a new BufferedReader object, passing the FileReader object as a parameter.
3. Use the readLine method of the BufferedReader object to read each line of the file.
4. Split each line by the delimiter (usually a comma) using the split method of the String class.
5. Parse the values as needed and store them in a data structure or object.
Here is an example code snippet that demonstrates how to read a .csv file in Java:
```
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadCSVFile {
public static void main(String[] args) {
String csvFile = "data.csv";
String line = "";
String delimiter = ",";
try (BufferedReader br = new BufferedReader(new FileReader(csvFile))) {
while ((line = br.readLine()) != null) {
String[] values = line.split(delimiter);
// Do something with the values
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
In this example, the code reads a file named "data.csv" and splits each line by a comma delimiter. You can modify the code to handle different delimiters or to parse the values in a different way depending on your specific use case.
阅读全文
相关推荐
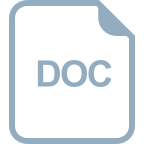
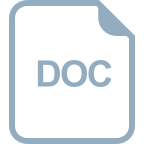
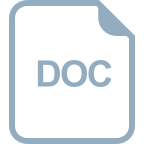

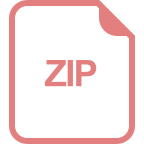
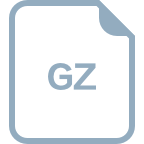
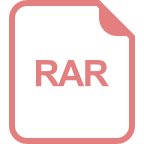
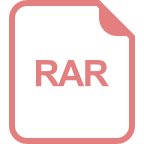
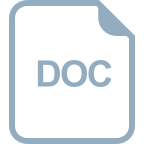
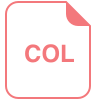
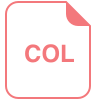
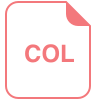
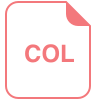
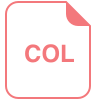
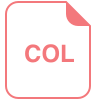



