解析一个 input.txt 文件中的价格数据,并计算总价格,将其平均价格写入到 output.txt 文件中。 例如:若 input.txt 文件内容如下: 则 output.txt 文件内容为: 商品列表: 电视机,2567 元/台 洗衣机,3262 元/台 冰箱,6573 元/台 则 output.txt 文件内容为: 所有商品总价格为 12402 元。用Java写
时间: 2024-02-12 22:07:36 浏览: 73
好的,我明白了你的问题。下面是Java实现的代码:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class PriceCalculator {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("input.txt"));
String line = null;
int totalPrice = 0;
int count = 0;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(",");
if (parts.length == 2) {
try {
int price = Integer.parseInt(parts[1].trim().replace("元/台", ""));
totalPrice += price;
count++;
} catch (NumberFormatException e) {
// 忽略不合法的价格
}
}
}
reader.close();
if (count > 0) {
int averagePrice = totalPrice / count;
FileWriter writer = new FileWriter("output.txt");
writer.write("所有商品总价格为 " + totalPrice + " 元。\n");
writer.write("平均价格为 " + averagePrice + " 元/台。");
writer.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
代码的主要思路是读取 input.txt 文件中的数据,解析每一行数据中的价格信息,累加总价格并统计商品数量,最后计算平均价格并将结果写入 output.txt 文件中。
需要注意的是,我们在解析价格信息时要先将字符串中的无关字符去掉,然后再将其转换为整数类型。在这个过程中可能会出现转换失败的情况,我们可以通过捕捉 NumberFormatException 异常来处理这种情况。
阅读全文
相关推荐
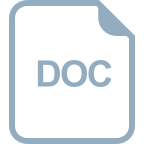
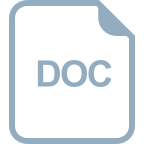
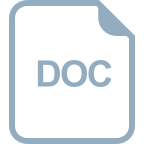





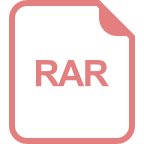
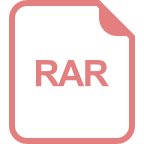
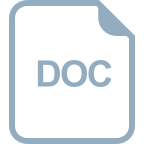
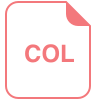
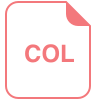
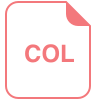
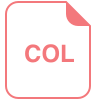
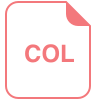
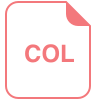
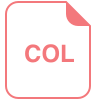
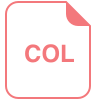