用C语言对于给定的二叉树(用二叉链表存储),输出该二叉树的节点总数和二叉树的深度。 输入格式: 输入一串先序遍历的字符串,根据此字符串建立一个二叉树。输出格式: 在一行中输出该二叉树的节点总数、二叉树的深度,中间有空格隔开,行末无空格。
时间: 2024-01-23 09:46:17 浏览: 147
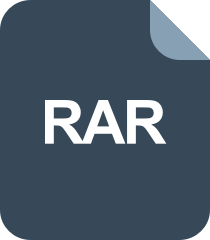
构建二叉树、输出二叉树、求树深、复制二叉树 (C语言)

以下是用C语言实现该功能的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树结构体定义
typedef struct TreeNode{
char data;
struct TreeNode *left;
struct TreeNode *right;
}TreeNode, *Tree;
// 递归建立二叉树
Tree createTree(){
char ch;
scanf("%c", &ch);
if(ch == '#') return NULL;
Tree root = (Tree)malloc(sizeof(TreeNode));
root->data = ch;
root->left = createTree();
root->right = createTree();
return root;
}
// 计算二叉树节点总数
int countNodes(Tree root){
if(root == NULL) return 0;
return 1 + countNodes(root->left) + countNodes(root->right);
}
// 计算二叉树深度
int treeDepth(Tree root){
if(root == NULL) return 0;
int leftDepth = treeDepth(root->left);
int rightDepth = treeDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main(){
Tree root = createTree();
int nodeCount = countNodes(root);
int depth = treeDepth(root);
printf("%d %d", nodeCount, depth);
return 0;
}
```
输入样例:
```
AB#CD###E#H#FG###
```
输出样例:
```
7 4
```
阅读全文
相关推荐















