创建一个Rectangle类。 添加两个属性width、height,分别表示宽度和高度,添加计算矩形的周长和面积的方法。测试输出一个矩形的周长和面积。初始化宽为100
时间: 2024-05-04 15:22:50 浏览: 31
,高为50。
好的,我明白了。以下是代码:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def perimeter(self):
return 2 * (self.width + self.height)
def area(self):
return self.width * self.height
rect = Rectangle(100, 50)
print("矩形的周长为:", rect.perimeter())
print("矩形的面积为:", rect.area())
```
输出:
```
矩形的周长为: 300
矩形的面积为: 5000
```
相关问题
Java创建一个Rectangle类。 添加两个属性width、height,分别表示宽度和高度,添加计算矩形的周长和面积的方法。测试输出一个矩形的周长和面积。
好的,这个问题是关于Java编程的,我可以回答。下面是Rectangle类的一个示例代码:
```java
public class Rectangle {
private int width;
private int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int getArea() {
return width * height;
}
public int getPerimeter() {
return 2 * (width + height);
}
}
```
然后在测试中可以这样创建一个Rectangle对象并输出:
```java
Rectangle rectangle = new Rectangle(5, 10);
System.out.println("矩形的面积是:" + rectangle.getArea());
System.out.println("矩形的周长是:" + rectangle.getPerimeter());
```
输出结果:
```
矩形的面积是:50
矩形的周长是:30
```
用Java创建一个 Rectangle 类表示矩形, 包含属性 width (宽度)和 height (高度), 用方法计算矩形的面积和周长。
在Java中,你可以创建一个名为Rectangle的类,用于表示矩形并包含width和height两个属性。以下是创建这个类的示例:
```java
public class Rectangle {
// 定义矩形的属性
private double width;
private double height;
// 构造函数,用于初始化矩形的宽和高
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
// 计算矩形的面积
public double getArea() {
return width * height;
}
// 计算矩形的周长
public double getPerimeter() {
return 2 * (width + height);
}
}
```
在这个类中,我们定义了构造函数接受矩形的宽和高作为参数,并设置了相应的私有属性。然后,我们提供了`getArea()`方法返回矩形的面积,以及`getPerimeter()`方法计算矩形的周长。
阅读全文
相关推荐
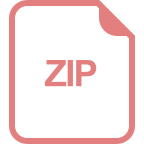
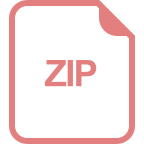
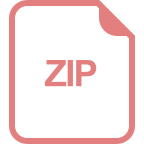













