ethercat样例
时间: 2023-07-26 08:03:23 浏览: 111
以下是一个使用 EtherCAT 通信的简单样例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <ethercat.h>
#define NUM_OF_ELMO_SLAVES 1
#define EC_TIMEOUTMON 500
int expectedWKC;
volatile int wkc;
ec_slave_config_t sc[NUM_OF_ELMO_SLAVES];
void check_domain_state(void) {
ec_domain_state_t ds;
ecrt_master_receive(master, EC_TIMEOUTRET, &ds);
ecrt_domain_state(domain[0], &ds);
if (ds.working_counter != wkc) {
printf("domain0: WC %u.\n", ds.working_counter);
wkc = ds.working_counter;
}
}
void cyclic_task(void) {
static int cnt = 0;
uint8_t *domain1_pd = ecrt_domain_data(domain[0]);
if (cnt > 1000) {
cnt = 0;
}
// Write data to PDOs
int32_t pos = cnt * 100;
int16_t torque = cnt * 10;
memcpy(domain1_pd + 0x00, &pos, sizeof(pos));
memcpy(domain1_pd + 0x04, &torque, sizeof(torque));
cnt++;
}
int main() {
printf("Starting Elmo Example\n");
// Initialize EtherCAT master
if (ecrt_master_open(&master, 0) != 0) {
printf("Failed to open EtherCAT master!\n");
return -1;
}
// Initialize EtherCAT slave configuration
if (ecrt_master_slave_config_init(master, NUM_OF_ELMO_SLAVES, slave_info) != 0) {
printf("Failed to initialize EtherCAT slave configuration!\n");
return -1;
}
// Get slave configuration
for (int i = 0; i < NUM_OF_ELMO_SLAVES; i++) {
if (!(sc[i] = ecrt_master_slave_config_find(master, i + 1))) {
printf("Failed to get slave %d configuration!\n", i + 1);
return -1;
}
}
// Create EtherCAT domain
if (!(domain = ecrt_master_create_domain(master))) {
printf("Failed to create EtherCAT domain!\n");
return -1;
}
// Configure slave PDOs
for (int i = 0; i < NUM_OF_ELMO_SLAVES; i++) {
if (ecrt_slave_config_pdos(sc[i], 0, slave_0_pdo_entries, slave_0_pdo_details) != NUM_OF_0_ENTRIES) {
printf("Failed to configure PDOs for slave %d!\n", i + 1);
return -1;
}
}
// Configure EtherCAT domain
if (ecrt_domain_reg_pdo_entry_list(domain[0], domain_regs) < 0) {
printf("Failed to configure EtherCAT domain!\n");
return -1;
}
// Activate master
if (ecrt_master_activate(master) != 0) {
printf("Failed to activate EtherCAT master!\n");
return -1;
}
printf("EtherCAT master activated.\n");
// Set expected working counter
expectedWKC = (ecrt_slave_config_max_data_size(sc[0]) / 2) + (ecrt_slave_config_watchdog_time(sc[0]) * 2) + 6;
// Start cyclic task
while (!done && (cyclic_count < 100000)) {
// Wait for next cycle
ecrt_master_receive(master, EC_TIMEOUTRET, &wkc);
check_domain_state();
if (wkc >= expectedWKC) {
cyclic_task();
ecrt_domain_queue(domain[0], ecrt_domain_data(domain[0]), PDO_SIZE);
} else {
printf("Working counter not expected.\n");
}
ecrt_master_send(master);
usleep(1000);
}
// Deactivate master
ecrt_master_deactivate(master);
ecrt_master_destroy(master);
printf("Master deactivated.\n");
return 0;
}
```
该样例中,使用了 EtherCAT 库的相关函数,例如 `ecrt_master_open()` 用于打开 EtherCAT 主站,`ecrt_master_slave_config_init()` 用于初始化从站配置等。在 `cyclic_task()` 函数中,将数据写入 PDO ,并在每个循环周期内执行。
需要注意的是,该样例中的从站配置、PDO 映射、PDO 数据等需要根据实际硬件进行修改。
阅读全文
相关推荐
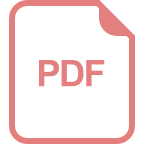
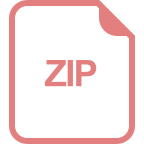
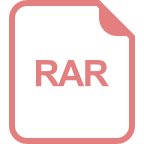
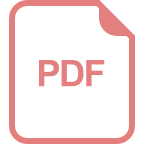
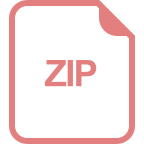
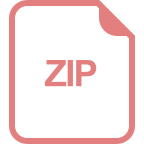
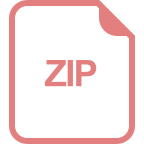
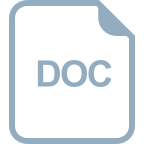
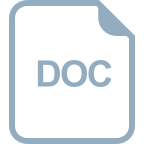
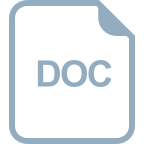
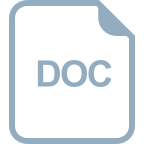
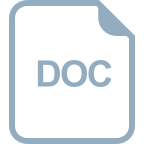


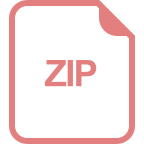