js 怎么替换html字符串中的css样式为变量
时间: 2024-05-15 09:16:22 浏览: 7
可以使用正则表达式和字符串替换函数来实现替换html字符串中的css样式为变量。
下面是一个示例代码:
```javascript
// 假设有一个html字符串
const htmlString = '<div class="box" style="background-color: #fff; width: 100px;"></div>';
// 定义一个变量,用于替换样式
const bgColor = '#f00';
// 使用正则表达式和字符串替换函数来替换样式
const newHtmlString = htmlString.replace(/style="([^"]*)"/, `style="$1background-color: ${bgColor};"`);
console.log(newHtmlString);
// 输出: <div class="box" style="background-color: #f00; width: 100px;"></div>
```
在上面的代码中,我们使用了正则表达式 `style="([^"]*)"` 来匹配 html 字符串中的样式字符串,然后使用字符串替换函数 `replace()` 来将匹配到的样式字符串替换为新的样式字符串,其中使用了变量 `bgColor` 来替换原来的背景颜色。
相关问题
模板字符串中如何添加css样式
在模板字符串中添加 CSS 样式,可以使用标签模板字符串(Tagged Template String)的方式,在模板字符串前面加上一个函数名,该函数将会接收模板字符串中的各个部分作为参数,我们可以在这个函数中对字符串进行处理和拼接,同时也可以添加 CSS 样式。
例如,下面的代码演示了如何使用标签模板字符串添加 CSS 样式:
```javascript
function addStyle(strings, ...values) {
let css = strings.join('');
values.forEach((value, i) => {
css += value + strings[i + 1];
})
return `<style>${css}</style>`;
}
const color = 'red';
const fontSize = '16px';
const style = addStyle`
p {
color: ${color};
font-size: ${fontSize};
}
`;
console.log(style);
```
输出结果为:
```html
<style>
p {
color: red;
font-size: 16px;
}
</style>
```
可以看到,我们在模板字符串中使用了 `${}` 语法来插入变量,同时在标签模板字符串函数 `addStyle` 中,将模板字符串的各个部分进行了拼接,最终返回一个包含 CSS 样式的字符串。
使HTML中等于字符串变量a的特定文本高亮
要在 HTML 中高亮等于字符串变量 `a` 的特定文本,可以使用 JavaScript 和 CSS 来实现。以下是一个例子:
```html
<p>This text contains the word "apple".</p>
<p>This text also contains the word "banana".</p>
<p>This text does not contain any fruit names.</p>
<script>
// 定义要高亮的字符串变量
const a = 'apple';
// 获取所有包含等于变量 a 的文本的元素
const highlightElements = document.querySelectorAll(':contains(' + a + ')');
// 遍历所有元素并添加高亮样式
highlightElements.forEach(el => {
el.innerHTML = el.innerHTML.replace(new RegExp(a, 'g'), '<span class="highlight">' + a + '</span>');
});
</script>
<style>
.highlight {
background-color: yellow;
font-weight: bold;
}
</style>
```
在上面的代码中,我们首先定义了要高亮的字符串变量 `a`。然后我们使用 JavaScript 获取了所有包含等于变量 `a` 的文本的元素,并使用正则表达式将所有等于变量 `a` 的文本替换为带有高亮样式的 HTML。最后,我们使用 CSS 定义了 "highlight" 类的样式,以实现高亮效果。
需要注意的是,这种方法仍然可能会影响性能,因为它需要遍历整个文档来查找匹配的文本。因此,如果您的文档非常大或包含大量匹配的文本,可能会导致性能问题。
相关推荐
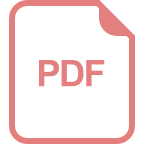
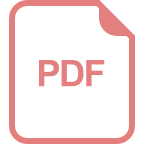
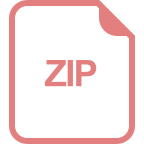












