pytorch建立lstm模型实现二分类预测模型
时间: 2023-11-12 11:06:15 浏览: 62
好的,下面是一个简单的基于 PyTorch 的 LSTM 二分类预测模型的代码示例:
```python
import torch
import torch.nn as nn
class LSTMClassifier(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_dim, output_dim, num_layers, bidirectional, dropout):
super().__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.lstm = nn.LSTM(embedding_dim, hidden_dim, num_layers=num_layers, bidirectional=bidirectional, dropout=dropout)
self.fc = nn.Linear(hidden_dim * 2 if bidirectional else hidden_dim, output_dim)
self.dropout = nn.Dropout(dropout)
def forward(self, text, text_lengths):
embedded = self.dropout(self.embedding(text))
packed_embedded = nn.utils.rnn.pack_padded_sequence(embedded, text_lengths.to('cpu'))
packed_output, (hidden, cell) = self.lstm(packed_embedded)
output, output_lengths = nn.utils.rnn.pad_packed_sequence(packed_output)
hidden = self.dropout(torch.cat((hidden[-2,:,:], hidden[-1,:,:]), dim=1) if self.lstm.bidirectional else hidden[-1,:,:])
return self.fc(hidden)
```
这是一个包含一个嵌入层、一个 LSTM 层和一个全连接层的模型。其中 `vocab_size` 是词汇表大小,`embedding_dim` 是词向量维度,`hidden_dim` 是 LSTM 隐藏层的维度,`output_dim` 是输出维度(即二分类的结果),`num_layers` 是 LSTM 层数,`bidirectional` 表示是否使用双向 LSTM,`dropout` 是 dropout 概率。
在 forward 方法中,我们首先将输入文本进行嵌入,然后使用 LSTM 层进行处理。由于输入文本长度不同,我们需要使用 `pack_padded_sequence` 和 `pad_packed_sequence` 函数对输入进行处理。最后,我们将 LSTM 层的输出通过全连接层得到最终的预测结果。
接下来,我们需要定义损失函数和优化器,并对模型进行训练和测试:
```python
import torch.optim as optim
# 定义模型和损失函数
model = LSTMClassifier(vocab_size, embedding_dim, hidden_dim, output_dim, num_layers, bidirectional, dropout).to(device)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters())
# 训练模型
for epoch in range(num_epochs):
for batch in train_iterator:
optimizer.zero_grad()
text, text_lengths = batch.text
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, batch.label)
loss.backward()
optimizer.step()
# 测试模型
def test_model(model, iterator):
correct = 0
total = 0
model.eval()
with torch.no_grad():
for batch in iterator:
text, text_lengths = batch.text
predictions = model(text, text_lengths).squeeze(1)
pred_labels = predictions.argmax(1)
correct += (pred_labels == batch.label).sum().item()
total += batch.batch_size
return correct / total
test_acc = test_model(model, test_iterator)
print(f'Test Accuracy: {test_acc:.3f}')
```
在训练过程中,我们首先将优化器的梯度清零,然后将输入文本和长度传递给模型,得到预测结果并计算损失。最后,我们使用反向传播更新模型参数。
在测试过程中,我们将模型设置为 eval 模式,然后对测试数据进行预测,并计算准确率。
这就是一个简单的基于 PyTorch 的 LSTM 二分类预测模型的实现。
相关推荐
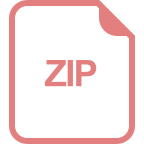
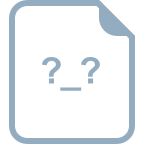
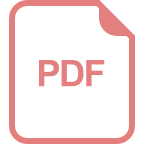
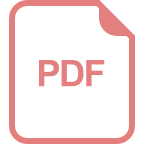
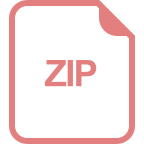
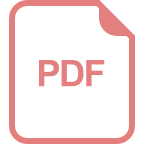
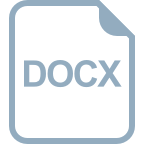
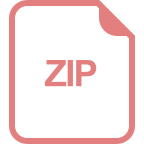
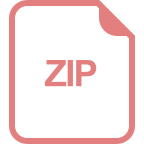
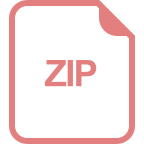