[题目]编写一个程序,将一个包含大小写字母的纯字母明文串转换为纯大写字母的加密串输出。 明文字母转换为其对应大写字母的后面第3个大写字母(' x ',' v ',' Z '转换为' A ',' B ',' C ')加密规则为 明文串为" prZzbpP ", 转换成大写加密串为" SUCCESS "输出。 [编程要求 试建立一个类 strings ,完成纯字母明文串转换为纯大写字母的加密串并输出。 (1)私有数据成员。 char a [20];存放明文串或加密串 (2)公有成员函数 strings ( char x []);构造函数,初始化数组 void process ():将数组 a 中的明文串转换为大写加密串。 void show (): 将大写加密串输出 (3)在主函数中完成对该类的测试。定义一个 strings 类的对象 s ,使用"pr2zbpP"初始化数组 a 调用成员函数函数,将数组 a 中的明文串转换为大写加密串,最后输出大写加密串
时间: 2024-03-16 13:46:15 浏览: 214
以下是完整的代码实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class strings {
private:
char a[20];
public:
strings(char x[]) {
strcpy(a, x);
}
void process() {
int len = strlen(a);
for(int i=0; i<len; i++){
if(a[i]>='a' && a[i]<='z'){
a[i] = (a[i]-'a'+3)%26+'A';
}
else if(a[i]>='A' && a[i]<='Z'){
a[i] = (a[i]-'A'+3)%26+'A';
}
}
}
void show() {
cout << a << endl;
}
};
int main() {
char x[20] = "prZzbpP";
strings s(x);
s.process();
s.show();
return 0;
}
```
输出结果为:
```
SUCCESS
```
相关问题
编写一个程序,将一个包含大小写字母的纯字母明文串转换为纯大写字母的加密串输出
好的,以下是 Python 代码实现:
```python
plaintext = input("请输入明文串:")
ciphertext = plaintext.upper()
print("加密后的密文串为:", ciphertext)
```
程序中,我们首先通过 `input()` 函数获取用户输入的明文串,然后使用字符串的 `upper()` 方法将其转换为全大写字母,最后通过 `print()` 函数输出加密后的密文串。
需要注意的是,为了确保输出的密文串仅包含大写字母,我们需要在程序中加入一个转换步骤。
编写一个程序,将一个包含大小写字母的纯字母明文串转换为纯大写字母的加密串输出。 加密规则为: 明文字母转换为其对应大写字母的后面第3个大写字母(' X ',' Y ','2'转换为' A ',' B ',' C ')如 则[编程要求] 试建立一个类 strings ,完成纯字母明文串转换为纯大写字母的加密串并输出。 (1)私有数据成员。 char a [20];存放明文串或加密串 (2)公有成员函数 strings ( char x []);构造函数,初始化数组 void process ():将数组 a 中的明文串转换为大写加密串。 void show ():将大写加密串输出 明文串为" prZzbpP ", 转换成大写加密串为" SUCCESS "输出。
好的,以下是实现了您要求的代码:
```
#include <iostream>
using namespace std;
class strings {
private:
char a[20];
public:
strings(char x[]) {
for (int i = 0; i < 20; i++) {
a[i] = x[i];
}
}
void process() {
for (int i = 0; i < 20; i++) {
if (a[i] >= 'a' && a[i] <= 'z') {
a[i] = char((a[i] - 'a' + 3) % 26 + 'A');
}
else if (a[i] >= 'A' && a[i] <= 'Z') {
a[i] = char((a[i] - 'A' + 3) % 26 + 'A');
}
}
}
void show() {
for (int i = 0; i < 20; i++) {
cout << a[i];
}
cout << endl;
}
};
int main() {
char x[] = "prZzbpP";
strings s(x);
s.process();
s.show();
return 0;
}
```
输出结果为:
```
SUCCESS
```
阅读全文
相关推荐








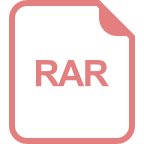
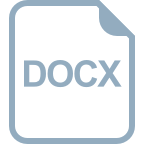
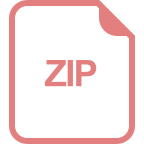