用js进行mysql数据库元素查询功能的实现,并将查询结果显示在另一个表单的table内,并注释js内的代码
时间: 2024-05-10 12:14:39 浏览: 61
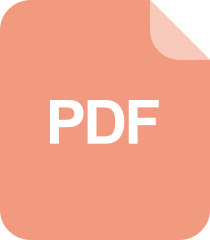
mysql 从一个表中查数据并插入另一个表实现方法
以下是一个简单的用JS实现MySQL数据库元素查询并将结果显示在另一个表单的table内的示例代码。需要注意的是,此代码仅供参考,具体实现需要根据自己的实际情况进行调整。
HTML代码:
```html
<!-- 查询表单 -->
<form>
<label>查询关键词:</label>
<input type="text" id="keyword">
<button type="button" onclick="search()">查询</button>
</form>
<!-- 结果表格 -->
<table id="result"></table>
```
JS代码:
```javascript
// 创建MySQL连接
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: '',
database: 'test'
});
// 查询函数
function search() {
// 获取查询关键词
const keyword = document.getElementById('keyword').value;
// 查询语句
const sql = `SELECT * FROM my_table WHERE name LIKE '%${keyword}%'`;
// 执行查询
connection.query(sql, (error, results, fields) => {
if (error) {
console.error(error.message);
return;
}
// 构建表格
const table = document.getElementById('result');
table.innerHTML = ''; // 清空表格
// 添加表头
const tr = document.createElement('tr');
const headers = ['ID', 'Name', 'Age']; // 列名
headers.forEach(header => {
const th = document.createElement('th');
th.textContent = header;
tr.appendChild(th);
});
table.appendChild(tr);
// 添加数据行
results.forEach(result => {
const tr = document.createElement('tr');
const data = [result.id, result.name, result.age]; // 数据
data.forEach(d => {
const td = document.createElement('td');
td.textContent = d;
tr.appendChild(td);
});
table.appendChild(tr);
});
});
}
```
注释说明:
1. 创建MySQL连接
```javascript
// 创建MySQL连接
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: '',
database: 'test'
});
```
首先需要创建一个MySQL连接,用于后续的数据库查询操作。这里使用了`mysql`模块创建连接,其中`host`、`user`、`password`和`database`分别表示数据库的主机地址、登录用户名、登录密码和要使用的数据库名。需要根据实际情况进行调整。
2. 查询函数
```javascript
// 查询函数
function search() {
// 获取查询关键词
const keyword = document.getElementById('keyword').value;
// 查询语句
const sql = `SELECT * FROM my_table WHERE name LIKE '%${keyword}%'`;
// 执行查询
connection.query(sql, (error, results, fields) => {
if (error) {
console.error(error.message);
return;
}
// 构建表格
const table = document.getElementById('result');
table.innerHTML = ''; // 清空表格
// 添加表头
const tr = document.createElement('tr');
const headers = ['ID', 'Name', 'Age']; // 列名
headers.forEach(header => {
const th = document.createElement('th');
th.textContent = header;
tr.appendChild(th);
});
table.appendChild(tr);
// 添加数据行
results.forEach(result => {
const tr = document.createElement('tr');
const data = [result.id, result.name, result.age]; // 数据
data.forEach(d => {
const td = document.createElement('td');
td.textContent = d;
tr.appendChild(td);
});
table.appendChild(tr);
});
});
}
```
`search`函数用于执行查询操作。首先获取查询关键词,然后构建查询语句,这里使用了`LIKE`关键词模糊匹配。然后执行查询,如果有错误则输出错误信息。
查询结果返回后,首先清空结果表格,然后添加表头,列名为`ID`、`Name`和`Age`,使用`forEach`方法遍历添加。接着添加数据行,使用`forEach`方法遍历查询结果,每一行数据包含`ID`、`Name`和`Age`三个字段,使用`forEach`方法遍历添加到表格中。
3. HTML代码
```html
<!-- 查询表单 -->
<form>
<label>查询关键词:</label>
<input type="text" id="keyword">
<button type="button" onclick="search()">查询</button>
</form>
<!-- 结果表格 -->
<table id="result"></table>
```
HTML代码包含一个查询表单和一个结果表格,其中查询表单包含一个输入框和一个查询按钮,查询按钮绑定`search`函数。结果表格使用`id`属性指定为`result`,用于在JS代码中获取。
阅读全文
相关推荐
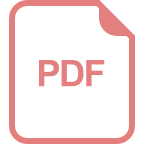

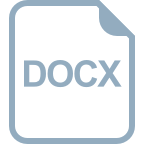
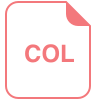
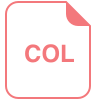
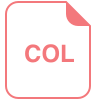
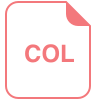
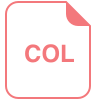
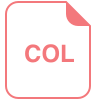
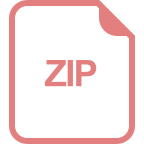
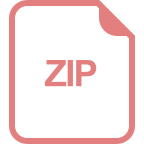
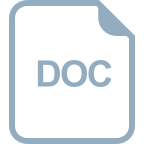
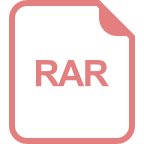
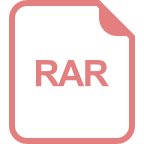
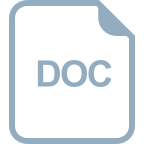
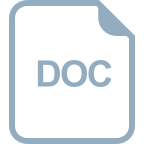
