在pyqt5中写代码建立一个可以增删改查商品信息的MySQL数据库,并为其创建含有一个信息显示表单和对应功能的按钮的主界面,描述实现过程及步骤,并给出代码注释
时间: 2024-02-19 10:59:03 浏览: 21
首先,需要安装 PyQt5 和 MySQL 数据库的 Python 连接器 PyMySQL,可以使用以下命令进行安装:
```
pip install PyQt5 PyMySQL
```
接着,我们需要创建一个 MySQL 数据库和一个商品信息表。可以使用以下 SQL 语句进行创建:
```sql
CREATE DATABASE IF NOT EXISTS product_db;
USE product_db;
CREATE TABLE IF NOT EXISTS product_info (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
price DECIMAL(10,2) NOT NULL,
quantity INT NOT NULL,
PRIMARY KEY (id)
);
```
接下来,我们可以开始编写 PyQt5 界面和对应的 MySQL 数据库操作代码。以下是一个示例代码,其中包含增删改查商品信息的功能:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton, QHBoxLayout, QVBoxLayout, QMessageBox, QTableWidget, QTableWidgetItem
import pymysql
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('商品信息管理系统')
self.resize(800, 600)
# 创建数据库连接
self.conn = pymysql.connect(
host='localhost',
port=3306,
user='root',
password='password',
db='product_db'
)
self.cur = self.conn.cursor()
# 创建控件
self.id_label = QLabel('ID:')
self.id_edit = QLineEdit()
self.name_label = QLabel('名称:')
self.name_edit = QLineEdit()
self.price_label = QLabel('价格:')
self.price_edit = QLineEdit()
self.quantity_label = QLabel('数量:')
self.quantity_edit = QLineEdit()
self.add_button = QPushButton('添加')
self.delete_button = QPushButton('删除')
self.update_button = QPushButton('修改')
self.search_button = QPushButton('查询')
self.table = QTableWidget()
self.table.setColumnCount(4)
self.table.setHorizontalHeaderLabels(['ID', '名称', '价格', '数量'])
# 添加控件到布局
input_layout = QHBoxLayout()
input_layout.addWidget(self.id_label)
input_layout.addWidget(self.id_edit)
input_layout.addWidget(self.name_label)
input_layout.addWidget(self.name_edit)
input_layout.addWidget(self.price_label)
input_layout.addWidget(self.price_edit)
input_layout.addWidget(self.quantity_label)
input_layout.addWidget(self.quantity_edit)
button_layout = QHBoxLayout()
button_layout.addWidget(self.add_button)
button_layout.addWidget(self.delete_button)
button_layout.addWidget(self.update_button)
button_layout.addWidget(self.search_button)
main_layout = QVBoxLayout()
main_layout.addLayout(input_layout)
main_layout.addLayout(button_layout)
main_layout.addWidget(self.table)
self.setLayout(main_layout)
# 绑定按钮事件
self.add_button.clicked.connect(self.add_product)
self.delete_button.clicked.connect(self.delete_product)
self.update_button.clicked.connect(self.update_product)
self.search_button.clicked.connect(self.search_product)
# 显示所有商品信息
self.show_all_products()
# 添加商品信息
def add_product(self):
name = self.name_edit.text()
price = self.price_edit.text()
quantity = self.quantity_edit.text()
try:
price = float(price)
quantity = int(quantity)
except ValueError:
QMessageBox.warning(self, '错误', '价格和数量必须为数字')
return
sql = 'INSERT INTO product_info (name, price, quantity) VALUES (%s, %s, %s)'
self.cur.execute(sql, (name, price, quantity))
self.conn.commit()
self.show_all_products()
# 删除商品信息
def delete_product(self):
id = self.id_edit.text()
if not id:
QMessageBox.warning(self, '错误', '请输入要删除的商品ID')
return
sql = 'DELETE FROM product_info WHERE id = %s'
self.cur.execute(sql, id)
self.conn.commit()
self.show_all_products()
# 修改商品信息
def update_product(self):
id = self.id_edit.text()
name = self.name_edit.text()
price = self.price_edit.text()
quantity = self.quantity_edit.text()
if not id:
QMessageBox.warning(self, '错误', '请输入要修改的商品ID')
return
try:
price = float(price)
quantity = int(quantity)
except ValueError:
QMessageBox.warning(self, '错误', '价格和数量必须为数字')
return
sql = 'UPDATE product_info SET name = %s, price = %s, quantity = %s WHERE id = %s'
self.cur.execute(sql, (name, price, quantity, id))
self.conn.commit()
self.show_all_products()
# 查询商品信息
def search_product(self):
id = self.id_edit.text()
name = self.name_edit.text()
sql = 'SELECT * FROM product_info'
if id:
sql += ' WHERE id = %s'
self.cur.execute(sql, id)
elif name:
sql += ' WHERE name LIKE %s'
self.cur.execute(sql, ('%' + name + '%',))
else:
self.cur.execute(sql)
rows = self.cur.fetchall()
self.table.setRowCount(len(rows))
for i, row in enumerate(rows):
for j, col in enumerate(row):
item = QTableWidgetItem(str(col))
self.table.setItem(i, j, item)
# 显示所有商品信息
def show_all_products(self):
self.cur.execute('SELECT * FROM product_info')
rows = self.cur.fetchall()
self.table.setRowCount(len(rows))
for i, row in enumerate(rows):
for j, col in enumerate(row):
item = QTableWidgetItem(str(col))
self.table.setItem(i, j, item)
# 关闭窗口时断开数据库连接
def closeEvent(self, event):
self.cur.close()
self.conn.close()
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在代码中,我们首先创建了一个 `MainWindow` 类作为主窗口,并在 `__init__` 方法中创建了控件和数据库连接。然后,我们在 `add_product`、`delete_product`、`update_product` 和 `search_product` 方法中实现了增删改查商品信息的功能,并在 `show_all_products` 方法中显示所有商品信息。最后,在 `closeEvent` 方法中断开数据库连接。
需要注意的是,在添加、删除和修改商品信息时,我们需要将价格和数量转换为浮点数和整数类型,否则会抛出 `ValueError` 异常。此外,在查询商品信息时,我们使用了 `LIKE` 操作符进行模糊查询,需要在查询字符串前后加上 `%` 符号。
相关推荐
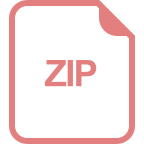
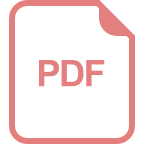
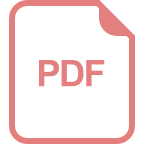














