用java编写酒店管理系统,要运用到GUI的知识点
时间: 2024-02-10 08:10:28 浏览: 130
好的,如果要在Java中编写一个GUI酒店管理系统,我们可以使用Swing或JavaFX来实现。下面是一个简单的GUI酒店管理系统的代码实现。
首先,我们需要创建一个主界面,用来显示酒店的信息和管理功能。可以使用JavaFX中的BorderPane布局来实现:
```java
public class HotelManagementSystem extends Application {
private HotelManager hotelManager;
private RoomManager roomManager;
private CustomerManager customerManager;
// other components
@Override
public void start(Stage primaryStage) throws Exception {
// initialize data managers
hotelManager = new HotelManager();
roomManager = new RoomManager();
customerManager = new CustomerManager();
// initialize components
// add components to the main pane
BorderPane root = new BorderPane();
root.setTop(createTopPane());
root.setCenter(createCenterPane());
root.setBottom(createBottomPane());
// create scene and show the stage
Scene scene = new Scene(root, 800, 600);
primaryStage.setTitle("Hotel Management System");
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
在主界面中,我们需要添加一些组件来显示酒店信息和管理功能,比如菜单栏、工具栏、表格等。可以使用JavaFX中的各种组件来实现。例如,可以使用TableView组件来显示酒店信息:
```java
private TableView<Hotel> hotelTable;
private Node createCenterPane() {
hotelTable = new TableView<>();
hotelTable.setItems(FXCollections.observableArrayList(hotelManager.getAllHotels()));
TableColumn<Hotel, Integer> idColumn = new TableColumn<>("ID");
idColumn.setCellValueFactory(new PropertyValueFactory<>("id"));
TableColumn<Hotel, String> nameColumn = new TableColumn<>("Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
TableColumn<Hotel, String> addressColumn = new TableColumn<>("Address");
addressColumn.setCellValueFactory(new PropertyValueFactory<>("address"));
hotelTable.getColumns().addAll(idColumn, nameColumn, addressColumn);
return hotelTable;
}
```
接下来,我们需要添加一些事件处理程序来实现各种管理功能,比如添加酒店、删除酒店、修改酒店信息等。可以使用JavaFX中的事件处理机制来实现。例如,可以使用Button组件来实现添加酒店的功能:
```java
private Node createBottomPane() {
Button addHotelButton = new Button("Add Hotel");
addHotelButton.setOnAction(event -> {
// show a dialog to input hotel information
HotelDialog dialog = new HotelDialog();
Optional<Hotel> result = dialog.showAndWait();
result.ifPresent(hotel -> {
hotelManager.addHotel(hotel);
hotelTable.getItems().add(hotel);
});
});
// other buttons
}
```
在按钮的事件处理程序中,我们可以弹出一个对话框来输入酒店信息,然后将酒店添加到数据管理器中并更新表格。对话框可以使用JavaFX中的Dialog组件来实现。
最后,我们需要将所有的组件和事件处理程序整合起来,并且在主方法中启动应用程序:
```java
public static void main(String[] args) {
launch(args);
}
```
通过这些代码的实现,我们可以在Java中编写一个GUI酒店管理系统,并且实现一些基本的管理功能。当然,这只是一个简单的示例,实际的酒店管理系统需要考虑更多的业务流程和数据管理,需要更加完善的代码实现。
阅读全文
相关推荐
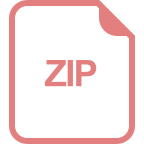
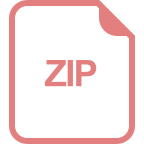
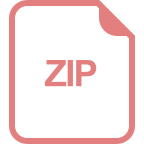
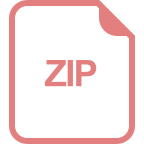
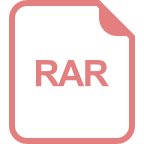
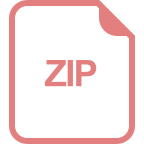
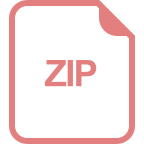
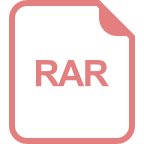
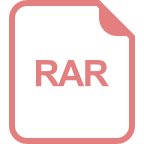
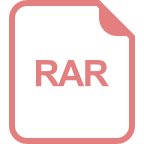
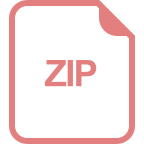
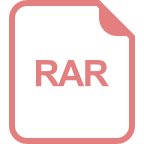
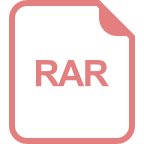
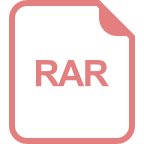
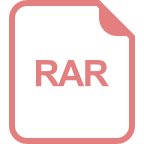
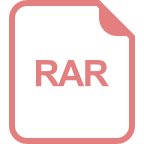
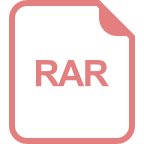