利用线性链表实现学生成绩管理系统,学生信息包含学号、姓名、成绩1、 成绩2、总分五项。
时间: 2023-08-31 07:53:04 浏览: 99
可以使用线性链表实现学生成绩管理系统,每个节点表示一个学生的信息,包含学号、姓名、成绩1、成绩2和总分五项。
首先,我们定义一个节点结构体,包含学生信息的各个字段:
```C++
struct Student {
int studentID;
string name;
float score1;
float score2;
float totalScore;
struct Student* next;
};
```
然后,我们可以定义一些操作函数来实现学生成绩管理系统的功能,比如添加学生信息、删除学生信息、查找学生信息等等。
以下是一个简单的示例代码,实现了添加学生信息和查找学生信息的功能:
```C++
#include <iostream>
using namespace std;
struct Student {
int studentID;
string name;
float score1;
float score2;
float totalScore;
struct Student* next;
};
struct Student* head = NULL;
// 添加学生信息
void addStudent(int studentID, string name, float score1, float score2) {
struct Student* newStudent = new Student;
newStudent->studentID = studentID;
newStudent->name = name;
newStudent->score1 = score1;
newStudent->score2 = score2;
newStudent->totalScore = score1 + score2;
newStudent->next = NULL;
if (head == NULL) {
head = newStudent;
} else {
struct Student* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newStudent;
}
}
// 查找学生信息
void findStudent(int studentID) {
struct Student* temp = head;
while (temp != NULL) {
if (temp->studentID == studentID) {
cout << "学生信息:" << endl;
cout << "学号:" << temp->studentID << endl;
cout << "姓名:" << temp->name << endl;
cout << "成绩1:" << temp->score1 << endl;
cout << "成绩2:" << temp->score2 << endl;
cout << "总分:" << temp->totalScore << endl;
return;
}
temp = temp->next;
}
cout << "未找到该学生信息!" << endl;
}
int main() {
addStudent(1, "张三", 90.5, 85.5);
addStudent(2, "李四", 80.5, 95.5);
addStudent(3, "王五", 88.5, 92.5);
findStudent(2); // 查找学号为2的学生信息
return 0;
}
```
以上代码实现了一个简单的学生成绩管理系统,你可以根据需要进行扩展,实现其他功能,比如删除学生信息、修改学生信息等等。
阅读全文
相关推荐



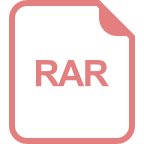

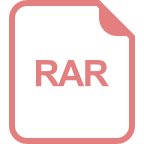

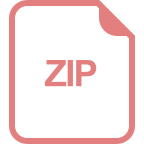
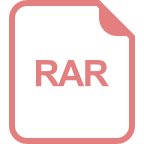








