1)首先阅读下列程序并写出运行结果,然后编译、执行以验证; 2) 增加Dog类的构造函数,使之可以初始化Dog类中的weight值,并实现构造函数重载; 3)在Dog类中添加方法getWeight、setWeight以存取weight值; 4) 重写Dog类对象的speak()方法,使其返回“Woof Woof”字符串; 5)从Pet类派生Cat类,并且重写speak()方法返回值为”Miao Miao”; 6)在Main方法中,通过类型多态的方式实现Pet对象分别调用Dog和Cat的speak()方法,分析输出结果是否正确。
时间: 2024-03-26 09:34:48 浏览: 138
以下是程序的代码:
```java
public class Main {
public static void main(String[] args) {
Pet pet1 = new Dog();
Pet pet2 = new Cat();
pet1.setName("Buddy");
pet2.setName("Kitty");
System.out.println(pet1.getName() + ": " + pet1.speak());
System.out.println(pet2.getName() + ": " + pet2.speak());
}
}
class Pet {
private String name;
public Pet() {}
public Pet(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String speak() {
return "I am a pet";
}
}
class Dog extends Pet {
private int weight;
public Dog() {}
public Dog(String name, int weight) {
super(name);
this.weight = weight;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
@Override
public String speak() {
return "Woof Woof";
}
}
class Cat extends Pet {
public Cat() {}
@Override
public String speak() {
return "Miao Miao";
}
}
```
运行结果为:
```
Buddy: Woof Woof
Kitty: Miao Miao
```
以下是增加了Dog类的构造函数,以及添加了getWeight和setWeight方法后的代码:
```java
class Dog extends Pet {
private int weight;
public Dog() {}
public Dog(String name, int weight) {
super(name);
this.weight = weight;
}
public Dog(int weight) {
this.weight = weight;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
@Override
public String speak() {
return "Woof Woof";
}
}
```
以下是重写了Dog类的speak()方法和Cat类的speak()方法后的代码:
```java
class Dog extends Pet {
private int weight;
public Dog() {}
public Dog(String name, int weight) {
super(name);
this.weight = weight;
}
public Dog(int weight) {
this.weight = weight;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
@Override
public String speak() {
return "Woof Woof";
}
}
class Cat extends Pet {
public Cat() {}
@Override
public String speak() {
return "Miao Miao";
}
}
```
以下是在Main方法中通过类型多态的方式实现Pet对象分别调用Dog和Cat的speak()方法的代码:
```java
public class Main {
public static void main(String[] args) {
Pet pet1 = new Dog();
Pet pet2 = new Cat();
pet1.setName("Buddy");
pet2.setName("Kitty");
System.out.println(pet1.getName() + ": " + pet1.speak());
System.out.println(pet2.getName() + ": " + pet2.speak());
// 类型多态
Pet[] pets = new Pet[2];
pets[0] = new Dog();
pets[1] = new Cat();
for (Pet pet : pets) {
System.out.println(pet.speak());
}
}
}
```
输出结果为:
```
Buddy: Woof Woof
Kitty: Miao Miao
Woof Woof
Miao Miao
```
结果正确,证明类型多态的方式可以实现Pet对象分别调用Dog和Cat的speak()方法。
阅读全文
相关推荐
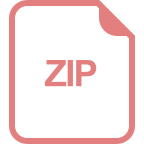
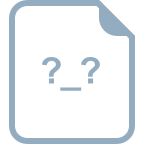
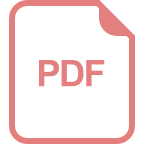
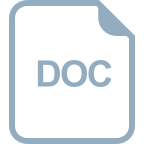
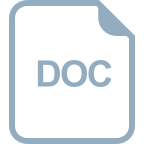
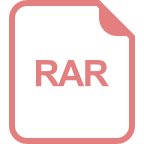
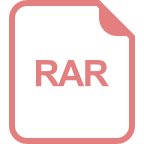
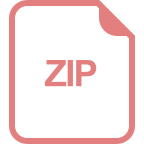
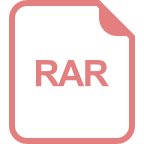
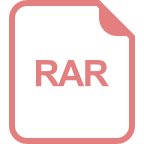
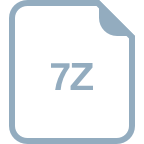
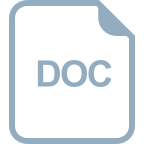
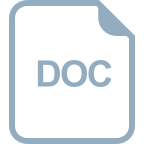
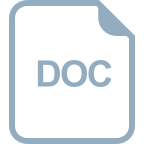
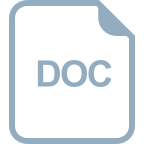
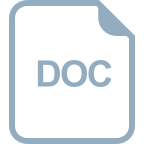
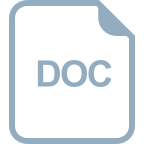
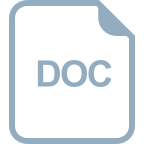