connection reset by peer: sock
时间: 2023-04-25 19:01:51 浏览: 182
et连接重置
这是一种网络错误,通常发生在客户端和服务器之间的通信过程中。它表示服务器已经关闭了与客户端的连接,可能是由于网络故障、服务器负载过高或其他原因导致的。这种错误通常需要重新连接或重新启动网络设备来解决。
相关问题
modbustcp Connection reset by peer: read
Connection reset by peer是一个常见的网络错误,它表示在TCP连接中对方(peer)意外关闭了连接。这种情况通常发生在服务器端关闭了连接,而客户端仍然试图读取数据。
在modbus TCP协议中,如果客户端在服务器端关闭连接后仍然尝试读取数据,就会出现Connection reset by peer错误。这通常是由于客户端在服务器关闭连接后没有正确处理连接关闭的情况导致的。
为了解决这个问题,你可以在客户端代码中添加异常处理来捕获Connection reset by peer错误,并在捕获到错误时进行相应的处理,例如重新建立连接或者退出程序。
以下是一个示例代码,演示了如何处理Connection reset by peer错误:
```python
import socket
# 创建socket连接
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('localhost', 502)
client_socket.connect(server_address)
try:
# 发送请求
request = b'\x01\x03\x00\x00\x00\x02\xC4\x0B'
client_socket.sendall(request)
# 接收响应
response = client_socket.recv(1024)
print("Received response:", response)
except ConnectionResetError:
print("Connection reset by peer. Reconnecting...")
# 重新建立连接
client_socket.close()
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(server_address)
finally:
# 关闭连接
client_socket.close()
```
在上述代码中,我们使用try-except语句来捕获ConnectionResetError异常,如果捕获到该异常,就会打印出"Connection reset by peer. Reconnecting..."的提示,并重新建立连接。无论是否捕获到异常,最后都会关闭连接。
ConnectionResetError: [Errno 104] Connection reset by peer什么意思
ConnectionResetError: [Errno 104] Connection reset by peer是一个socket错误,意味着远程主机强制关闭了一个现有的连接。这通常是由于以下原因之一引起的:
- 远程主机已经关闭了连接,但本地主机仍在发送数据。
- 本地主机已经关闭了连接,但远程主机仍在发送数据。
- 在连接过程中出现了网络故障,例如超时或连接中断。
如果您遇到此错误,可以尝试以下解决方法:
- 检查您的网络连接是否正常。
- 检查您的代码是否正确关闭了连接。
- 尝试增加socket超时时间,以避免连接超时。
- 尝试使用更可靠的协议,例如TCP,以确保连接的稳定性。
以下是一个示例代码,演示了如何处理ConnectionResetError错误:
```python
import socket
try:
# 进行socket连接
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('www.example.com', 80))
# 发送数据
s.sendall(b'GET / HTTP/1.1\r\nHost: www.example.com\r\n\r\n')
# 接收数据
data = s.recv(1024)
# 关闭连接
s.close()
except socket.error as e:
if e.errno == socket.errno.ECONNRESET:
print("Connection reset by peer")
else:
print("Socket error: ", e)
```
阅读全文
相关推荐
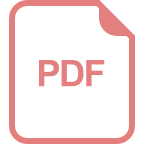
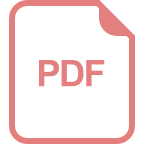
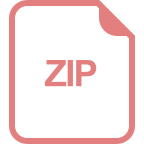



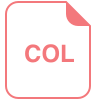
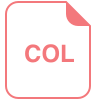
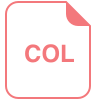
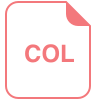
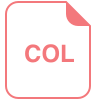
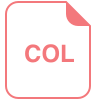
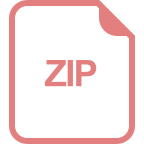