用C++语言编写一个函数,输出数组中非零元素的个数
时间: 2023-05-19 20:07:02 浏览: 132
可以使用以下代码实现:
```c
int countNonZero(int arr[], int n) {
int count = 0;
for (int i = 0; i < n; i++) {
if (arr[i] != 0) {
count++;
}
}
return count;
}
```
其中,arr[] 表示输入的数组,n 表示数组的长度。该函数会遍历整个数组,每当遇到一个非零元素时,计数器 count 就会加一。最终返回 count 的值,即非零元素的个数。
相关问题
用C++语言编写一个函数,输出二维数组中非零元素的个数
好的,以下是用C语言编写的函数,可以输出二维数组中非零元素的个数:
```c
int countNonZero(int arr[][N], int row, int col) {
int count = 0;
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (arr[i][j] != 0) {
count++;
}
}
}
return count;
}
```
其中,`arr`是一个二维数组,`row`和`col`分别表示数组的行数和列数。函数会遍历整个数组,统计非零元素的个数,并返回结果。
希望这个函数能够帮到你!
用C++编写一个函数,实现数组中元素逆序
可以使用以下代码实现:
```
void reverseArray(int arr[], int n)
{
int i, temp;
for (i = 0; i < n / 2; i++)
{
temp = arr[i];
arr[i] = arr[n - i - 1];
arr[n - i - 1] = temp;
}
}
```
相关推荐
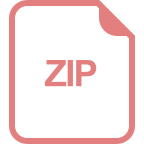












