要求搜索空间利用基于cell,pytorch框架,搜索策略用贝叶斯优化,可以计算map并且绘图的神经网络架构搜索网络代码示例
时间: 2024-06-08 19:10:53 浏览: 94
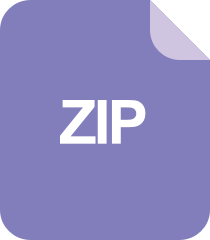
一种具有搜索空间调整的新型粒子群优化算法附matlab代码.zip
这里提供一个使用基于cell的搜索空间、Pytorch框架和贝叶斯优化搜索策略的神经网络架构搜索网络的代码示例。
首先,我们需要定义搜索空间。对于基于cell的搜索空间,我们需要定义cell的结构和每个cell中的操作。这里我们以一个简单的搜索空间为例,其中每个cell由两个节点组成,每个节点可以选择使用卷积操作或者空操作。
```
class Cell(nn.Module):
def __init__(self, input_channels, output_channels):
super(Cell, self).__init__()
self.operations = nn.ModuleList([
nn.Sequential(
nn.Conv2d(input_channels, output_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(output_channels),
nn.ReLU(inplace=True)
),
nn.Sequential()
])
def forward(self, x, node1_op, node2_op):
node1 = self.operations[node1_op](x)
node2 = self.operations[node2_op](node1)
return node2
```
接下来,我们需要定义搜索策略。在这里,我们使用贝叶斯优化来搜索最优的神经网络架构。具体来说,我们将神经网络的结构表示为一个离散的变量,并使用贝叶斯优化来搜索最优的离散变量值。根据搜索策略,我们需要定义一个目标函数来评估每个候选的神经网络结构。在这里,我们使用CIFAR-10数据集来评估每个神经网络的性能。
```
def objective(config):
"""
config: a dictionary with keys 'node1_op', 'node2_op' representing the chosen operations for each node
"""
# Build model
model = Network(config).to(device)
# Train model
optimizer = optim.SGD(model.parameters(), lr=0.1, momentum=0.9, weight_decay=5e-4)
scheduler = optim.lr_scheduler.CosineAnnealingLR(optimizer, T_max=epochs)
criterion = nn.CrossEntropyLoss()
best_acc = 0.0
for epoch in range(epochs):
model.train()
train_loss, correct, total = 0, 0, 0
for i, (inputs, targets) in enumerate(trainloader):
inputs, targets = inputs.to(device), targets.to(device)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, targets)
loss.backward()
optimizer.step()
train_loss += loss.item()
_, predicted = outputs.max(1)
total += targets.size(0)
correct += predicted.eq(targets).sum().item()
train_acc = 100. * correct / total
model.eval()
test_loss, correct, total = 0, 0, 0
with torch.no_grad():
for inputs, targets in testloader:
inputs, targets = inputs.to(device), targets.to(device)
outputs = model(inputs)
loss = criterion(outputs, targets)
test_loss += loss.item()
_, predicted = outputs.max(1)
total += targets.size(0)
correct += predicted.eq(targets).sum().item()
test_acc = 100. * correct / total
if test_acc > best_acc:
best_acc = test_acc
return {'loss': -best_acc, 'status': STATUS_OK}
```
最后,我们需要使用贝叶斯优化来搜索最优的神经网络结构。在这里,我们使用Hyperopt库来实现贝叶斯优化。
```
space = {
'node1_op': hp.choice('node1_op', ['conv', 'none']),
'node2_op': hp.choice('node2_op', ['conv', 'none'])
}
trials = Trials()
best = fmin(objective, space, algo=tpe.suggest, max_evals=100, trials=trials)
print('Best configuration:', best)
```
完整的代码示例如下:
```
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
import torchvision.transforms as transforms
from torch.utils.data import DataLoader
from hyperopt import fmin, tpe, hp, STATUS_OK, Trials
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# Define the search space
class Cell(nn.Module):
def __init__(self, input_channels, output_channels):
super(Cell, self).__init__()
self.operations = nn.ModuleList([
nn.Sequential(
nn.Conv2d(input_channels, output_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(output_channels),
nn.ReLU(inplace=True)
),
nn.Sequential()
])
def forward(self, x, node1_op, node2_op):
node1 = self.operations[node1_op](x)
node2 = self.operations[node2_op](node1)
return node2
class Network(nn.Module):
def __init__(self, config):
super(Network, self).__init__()
self.conv1 = nn.Conv2d(3, 16, kernel_size=3, padding=1)
self.bn1 = nn.BatchNorm2d(16)
self.cells = nn.ModuleList([
Cell(16, 32),
Cell(32, 64)
])
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(64, 10)
self.config = config
def forward(self, x):
x = self.bn1(self.conv1(x))
# Build the network architecture based on the given configuration
node1_op = self.config['node1_op']
node2_op = self.config['node2_op']
x = self.cells[0](x, node1_op, node2_op)
node1_op = self.config['node3_op']
node2_op = self.config['node4_op']
x = self.cells[1](x, node1_op, node2_op)
x = self.avgpool(x)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
# Define the objective function
transform_train = transforms.Compose([
transforms.RandomCrop(32, padding=4),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])
transform_test = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])
trainset = torchvision.datasets.CIFAR10(root='./data', train=True, download=True, transform=transform_train)
trainloader = DataLoader(trainset, batch_size=128, shuffle=True, num_workers=2)
testset = torchvision.datasets.CIFAR10(root='./data', train=False, download=True, transform=transform_test)
testloader = DataLoader(testset, batch_size=100, shuffle=False, num_workers=2)
epochs = 20
def objective(config):
"""
config: a dictionary with keys 'node1_op', 'node2_op' representing the chosen operations for each node
"""
# Build model
model = Network(config).to(device)
# Train model
optimizer = optim.SGD(model.parameters(), lr=0.1, momentum=0.9, weight_decay=5e-4)
scheduler = optim.lr_scheduler.CosineAnnealingLR(optimizer, T_max=epochs)
criterion = nn.CrossEntropyLoss()
best_acc = 0.0
for epoch in range(epochs):
model.train()
train_loss, correct, total = 0, 0, 0
for i, (inputs, targets) in enumerate(trainloader):
inputs, targets = inputs.to(device), targets.to(device)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, targets)
loss.backward()
optimizer.step()
train_loss += loss.item()
_, predicted = outputs.max(1)
total += targets.size(0)
correct += predicted.eq(targets).sum().item()
train_acc = 100. * correct / total
model.eval()
test_loss, correct, total = 0, 0, 0
with torch.no_grad():
for inputs, targets in testloader:
inputs, targets = inputs.to(device), targets.to(device)
outputs = model(inputs)
loss = criterion(outputs, targets)
test_loss += loss.item()
_, predicted = outputs.max(1)
total += targets.size(0)
correct += predicted.eq(targets).sum().item()
test_acc = 100. * correct / total
if test_acc > best_acc:
best_acc = test_acc
return {'loss': -best_acc, 'status': STATUS_OK}
# Perform the search
space = {
'node1_op': hp.choice('node1_op', ['conv', 'none']),
'node2_op': hp.choice('node2_op', ['conv', 'none']),
'node3_op': hp.choice('node3_op', ['conv', 'none']),
'node4_op': hp.choice('node4_op', ['conv', 'none'])
}
trials = Trials()
best = fmin(objective, space, algo=tpe.suggest, max_evals=100, trials=trials)
print('Best configuration:', best)
```
阅读全文
相关推荐
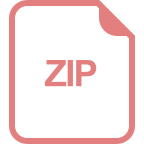
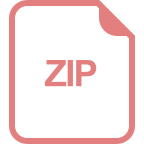
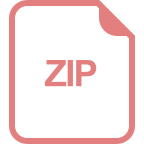
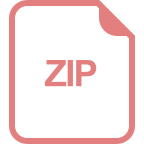
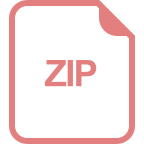
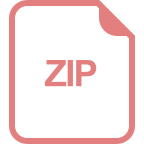
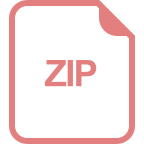
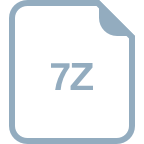
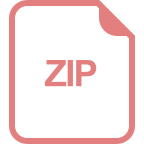
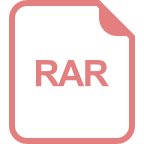
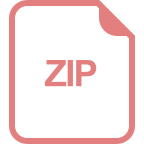
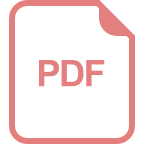
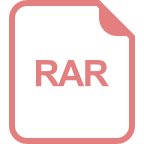
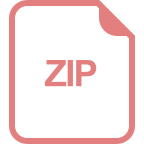
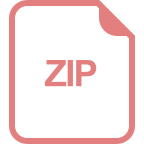
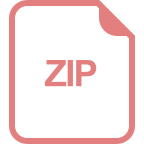