follows=input().split() sorted_follows=sorted(follows[1:]) m=int(input()) likes={} results=[] for i in range(m): like=input().split() likes[like[0]]=int(like[1]) average_like=sum(likes.values())/m for k,v in likes.items(): if v>average_like: l=0 r=len(sorted_follows)-1 flag=False while l<=r: mid=(l+r)//2 if k==sorted_follows[mid]: flag=True break elif k > sorted_follows[mid]: l=mid+1 elif k < sorted_follows[mid]: r=mid-1 if flag==False: results.append(k) if results: for name in sorted(results): print(name) else: print("Bing Mei You")
时间: 2024-04-28 13:25:40 浏览: 14
这是一段 Python 代码,它的作用是输入一个关注列表和一些用户的点赞数,然后输出所有点赞数高于平均值且不在关注列表中的用户名称,与之前的代码相比,这段代码增加了一个二分查找的过程来判断用户是否在关注列表中。
具体地,代码首先读取输入的关注列表和用户点赞数,然后计算所有用户点赞数的平均值。接着,它遍历所有用户的点赞数,如果某个用户的点赞数高于平均值且不在关注列表中,则将该用户的名称加入结果列表中。在加入之前,程序会对关注列表进行排序,并使用二分查找来判断该用户是否在关注列表中。
最后,如果结果列表不为空,则按名称排序并输出所有符合条件的用户名称;否则输出 "Bing Mei You"。
相关问题
3.假如我们有名为df的Series类型的数据,但是df中的标签不是按顺序排列的,如果我们想要令df的标签按升序进行排列,我们不应 该用以下哪一代码? A.Sorted_df= df.sort_values() B.sorted_df= df.sort_values (ascending=False) C.sorted_df= df.sort_values (ascending=True) D.sorted_df= df.reindex()
正确答案是 B. sorted_df = df.sort_values(ascending=False)。
sort_values() 方法可以用来对 Series 或 DataFrame 中的数值进行排序,按照指定的排序方式进行排序,默认是按照升序排序。如果想要按照降序排序,应该将参数 `ascending` 设置为 `False`,而不是 `True`。因此,选项 B 中的代码是错误的。
正确的选项是 A、C 和 D,它们可以按照升序对 df 的标签进行排序。其中:
- A. Sorted_df = df.sort_values() 可以按照升序对 df 进行排序,返回一个新的按标签排序后的 Series;
- C. sorted_df = df.sort_values(ascending=True) 和 A 的效果相同,可以按照升序对 df 进行排序;
- D. sorted_df = df.reindex() 可以对 df 进行重新索引,如果不指定索引顺序,则会按照升序对标签进行排序。
二、多项选择题(共25题,每题有至少一个正确选项3.假如我们有名为df的Series类型的数据,但是df中的标签不是按顺序排列的,如果我们想要令df的标签按升序进行排列,我们不应 该用以下哪一代码? A.Sorted_df= df.sort_values () B.sorted_df= df.sort_values (ascending=False) C.sorted_df= df.sort_values (ascending=True) D.sorted_df= df.reindex()
正确答案是 B. `sorted_df = df.sort_values(ascending=False)`。
`sort_values()` 方法可以用来对 Series 或 DataFrame 中的数值进行排序,按照指定的排序方式进行排序,默认是按照升序排序。如果想要按照降序排序,应该将参数 `ascending` 设置为 `False`,而不是 `True`。因此,选项 B 中的代码是错误的。
正确的选项是 A、C 和 D,它们可以按照升序对 df 的标签进行排序。其中:
- A. `sorted_df = df.sort_values()` 可以按照升序对 df 进行排序,返回一个新的按标签排序后的 Series;
- C. `sorted_df = df.sort_values(ascending=True)` 和 A 的效果相同,可以按照升序对 df 进行排序;
- D. `sorted_df = df.reindex()` 可以对 df 进行重新索引,如果不指定索引顺序,则会按照升序对标签进行排序。
相关推荐
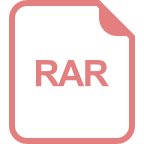
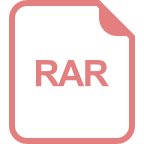
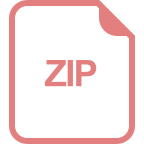














