用数据分析python写一个图书管理系统
时间: 2023-07-18 07:07:18 浏览: 103
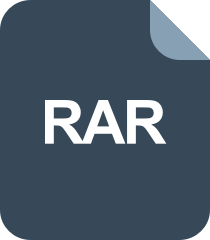
基于python的图书管理系统

好的,以下是一个简单的图书管理系统的示例代码:
```python
import pandas as pd
class Book:
def __init__(self, title, author, genre, year):
self.title = title
self.author = author
self.genre = genre
self.year = year
def __repr__(self):
return f"{self.title} by {self.author}"
class Library:
def __init__(self):
self.books = pd.DataFrame(columns=["Title", "Author", "Genre", "Year"])
def add_book(self, book):
self.books = self.books.append({
"Title": book.title,
"Author": book.author,
"Genre": book.genre,
"Year": book.year
}, ignore_index=True)
def remove_book(self, title):
self.books = self.books[self.books.Title != title]
def search(self, title=None, author=None, genre=None, year=None):
results = self.books
if title:
results = results[results.Title == title]
if author:
results = results[results.Author == author]
if genre:
results = results[results.Genre == genre]
if year:
results = results[results.Year == year]
return results
def display_books(self):
print(self.books)
```
此处使用pandas库创建一个DataFrame来存储图书信息,每本图书使用Book类来表示。Library类包括添加、删除、搜索和显示图书的方法。
我们现在可以创建一个Library对象并添加一些书籍:
```python
library = Library()
book1 = Book("The Great Gatsby", "F. Scott Fitzgerald", "Fiction", 1925)
book2 = Book("To Kill a Mockingbird", "Harper Lee", "Fiction", 1960)
book3 = Book("Pride and Prejudice", "Jane Austen", "Romance", 1813)
library.add_book(book1)
library.add_book(book2)
library.add_book(book3)
library.display_books()
```
输出结果应为:
```
Title Author Genre Year
0 The Great Gatsby F. Scott Fitzgerald Fiction 1925
1 To Kill a Mockingbird Harper Lee Fiction 1960
2 Pride and Prejudice Jane Austen Romance 1813
```
我们可以使用search方法来查找书籍:
```python
results = library.search(title="The Great Gatsby", author="F. Scott Fitzgerald")
print(results)
```
输出结果应为:
```
Title Author Genre Year
0 The Great Gatsby F. Scott Fitzgerald Fiction 1925
```
最后,我们可以使用remove_book方法删除一本书:
```python
library.remove_book("To Kill a Mockingbird")
library.display_books()
```
输出结果应为:
```
Title Author Genre Year
0 The Great Gatsby F. Scott Fitzgerald Fiction 1925
2 Pride and Prejudice Jane Austen Romance 1813
```
这是一个简单的图书管理系统,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐

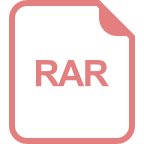
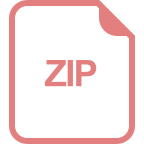
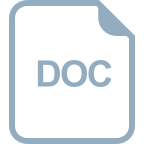







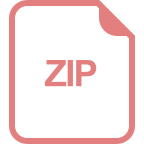
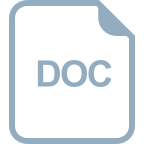
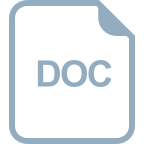

