vue+springboot+element回显MySQL文件和表单数据的所有代码应该怎么写
时间: 2023-06-18 10:03:09 浏览: 184
下面是一个简单的示例,展示如何使用Vue和Spring Boot实现回显MySQL文件和表单数据。
1. 配置MySQL数据库连接
在Spring Boot的application.properties文件中配置MySQL数据库连接信息,例如:
```
spring.datasource.url=jdbc:mysql://localhost:3306/db_name
spring.datasource.username=username
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
2. 创建MySQL表
在MySQL数据库中创建一个表,用于存储文件和表单数据,例如:
```
CREATE TABLE `file_data` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`file_name` varchar(255) DEFAULT NULL,
`file_data` longblob,
`form_data` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
3. 编写Spring Boot后端代码
创建一个Spring Boot的RestController,用于处理HTTP请求并从MySQL数据库中检索文件和表单数据。下面是一个简单的示例:
```
@RestController
@RequestMapping("/api")
public class FileDataController {
@Autowired
private FileDataRepository fileDataRepository;
@GetMapping("/file-data")
public List<FileData> getFileData() {
return fileDataRepository.findAll();
}
@PostMapping("/file-data")
public void saveFileData(@RequestBody FileData fileData) {
fileDataRepository.save(fileData);
}
}
```
4. 编写Vue前端代码
使用Vue和Element UI创建一个简单的表单,用于上传文件和提交表单数据。下面是一个简单的示例:
```
<template>
<el-form :model="form" ref="form" label-width="100px">
<el-form-item label="Upload File">
<el-upload
class="upload-demo"
:action="uploadUrl"
:on-success="handleFileUploadSuccess"
:before-upload="beforeFileUpload"
:show-file-list="false">
<el-button slot="trigger" size="small" type="primary">Select File</el-button>
</el-upload>
</el-form-item>
<el-form-item label="Form Data">
<el-input v-model="form.formData"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('form')">Submit</el-button>
</el-form-item>
</el-form>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
form: {
formData: '',
file: null
},
uploadUrl: '/api/file-data'
}
},
methods: {
beforeFileUpload(file) {
this.form.file = file
return true
},
handleFileUploadSuccess(response) {
console.log(response)
},
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
let formData = new FormData()
formData.append('formData', this.form.formData)
formData.append('file', this.form.file)
axios.post(this.uploadUrl, formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
}).then(response => {
console.log(response)
}).catch(error => {
console.log(error)
})
} else {
console.log('error submit!!')
return false
}
})
}
}
}
</script>
```
5. 创建FileData实体类和Repository
创建一个FileData实体类,用于映射MySQL表中的数据。创建一个FileDataRepository,用于从MySQL数据库中检索数据。下面是一个简单的示例:
```
@Entity
@Table(name = "file_data")
public class FileData {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "file_name")
private String fileName;
@Lob
@Column(name = "file_data")
private byte[] fileData;
@Column(name = "form_data")
private String formData;
// getters and setters
}
@Repository
public interface FileDataRepository extends JpaRepository<FileData, Long> {
}
```
以上就是一个简单的示例,展示了如何使用Vue和Spring Boot实现回显MySQL文件和表单数据的代码。
阅读全文
相关推荐
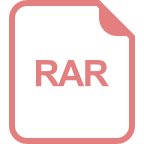
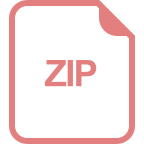
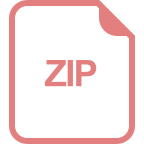
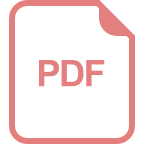
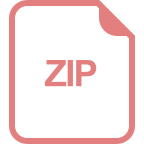
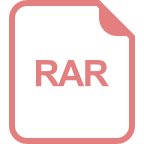
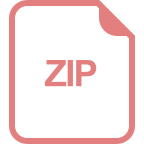
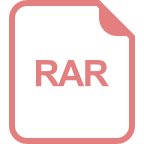
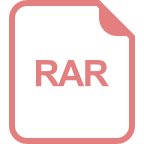
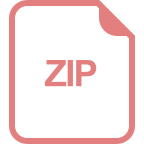
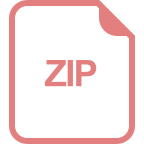
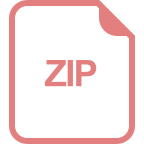
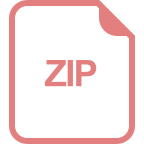
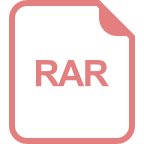
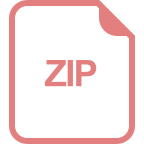
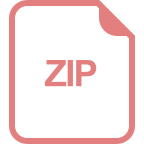
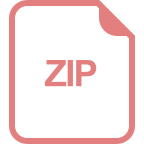
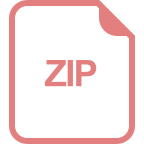
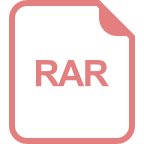
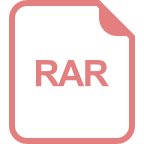