用micpython写一个ILI9342C驱动程序
时间: 2023-11-16 18:03:52 浏览: 241
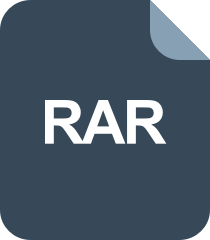
ILI9342.rar_9342.com_ILI9342C 中文_fmc ili9342_ili9342 spi_海思 ili9

ILI9342C是一款常见的TFT LCD控制器芯片,它可以通过SPI接口与微控制器通信。以下是一个基本的Micropython ILI9342C驱动程序的示例:
```python
from micropython import const
import framebuf
import time
# ILI9342C命令
ILI_NOP = const(0x00)
ILI_SWRESET = const(0x01)
ILI_RDDID = const(0x04)
ILI_RDDST = const(0x09)
# 控制寄存器
ILI_SLPIN = const(0x10)
ILI_SLPOUT = const(0x11)
ILI_PTLON = const(0x12)
ILI_NORON = const(0x13)
# 显示窗口
ILI_CASET = const(0x2A)
ILI_PASET = const(0x2B)
ILI_RAMWR = const(0x2C)
# 颜色格式
ILI_COLMOD = const(0x3A)
class ILI9342C(framebuf.FrameBuffer):
def __init__(self, spi, cs, dc, rst=None, width=240, height=320):
self.spi = spi
self.cs = cs
self.dc = dc
self.rst = rst
self.width = width
self.height = height
self.buffer = bytearray(width * height * 2)
super().__init__(self.buffer, width, height, framebuf.RGB565)
self.reset()
self.init()
def reset(self):
if self.rst != None:
self.rst.value(0)
time.sleep_ms(50)
self.rst.value(1)
time.sleep_ms(50)
def init(self):
# 初始化步骤1
self.write_cmd(ILI_SWRESET)
time.sleep_ms(120)
# 初始化步骤2
self.write_cmd(0xEF)
self.write_data(0x03)
self.write_data(0x80)
self.write_data(0x02)
# 初始化步骤3
self.write_cmd(0xCF)
self.write_data(0x00)
self.write_data(0xC1)
self.write_data(0x30)
# 初始化步骤4
self.write_cmd(0xED)
self.write_data(0x64)
self.write_data(0x03)
self.write_data(0x12)
self.write_data(0x81)
# 初始化步骤5
self.write_cmd(0xE8)
self.write_data(0x85)
self.write_data(0x00)
self.write_data(0x78)
# 初始化步骤6
self.write_cmd(0xCB)
self.write_data(0x39)
self.write_data(0x2C)
self.write_data(0x00)
self.write_data(0x34)
self.write_data(0x02)
# 初始化步骤7
self.write_cmd(0xF7)
self.write_data(0x20)
# 初始化步骤8
self.write_cmd(0xEA)
self.write_data(0x00)
self.write_data(0x00)
# 初始化步骤9
self.write_cmd(ILI_COLMOD)
self.write_data(0x55)
# 初始化步骤10
self.write_cmd(ILI_MADCTL)
self.write_data(0x48)
# 初始化步骤11
self.write_cmd(ILI_SLPOUT)
time.sleep_ms(120)
# 初始化步骤12
self.write_cmd(ILI_DISPON)
def write_cmd(self, cmd):
self.dc.value(0) # 发送命令
self.cs.value(0) # 选中芯片
self.spi.write(bytearray([cmd]))
self.cs.value(1) # 取消片选
def write_data(self, data):
self.dc.value(1) # 发送数据
self.cs.value(0) # 选中芯片
self.spi.write(bytearray([data]))
self.cs.value(1) # 取消片选
def show(self):
self.write_cmd(ILI_CASET)
self.write_data(0)
self.write_data(0)
self.write_data(self.width >> 8)
self.write_data(self.width & 0xFF)
self.write_cmd(ILI_PASET)
self.write_data(0)
self.write_data(0)
self.write_data(self.height >> 8)
self.write_data(self.height & 0xFF)
self.write_cmd(ILI_RAMWR)
self.cs.value(1)
self.dc.value(1)
self.cs.value(0)
self.spi.write(self.buffer)
self.cs.value(1)
```
使用此驱动程序,您可以像下面这样在Micropython中初始化并使用ILI9342C:
```python
import machine
import ili9342c
spi = machine.SPI(1, baudrate=20000000, polarity=0, phase=0)
cs = machine.Pin(15, machine.Pin.OUT)
dc = machine.Pin(2, machine.Pin.OUT)
rst = machine.Pin(0, machine.Pin.OUT)
display = ili9342c.ILI9342C(spi, cs, dc, rst)
display.fill(0xFFFF)
display.show()
```
在上面的示例中,我们使用SPI1接口,SCLK、MOSI和MISO分别连接到IO14、IO13和IO12引脚。片选引脚连接到IO15,数据/命令(DC)引脚连接到IO2,复位(RST)引脚连接到IO0。连接后,我们可以使用`fill()`填充显示器并使用`show()`显示图像。
阅读全文
相关推荐
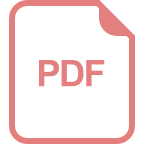















