用python一个测试sata ssd的python脚本,测试步骤如下: 1、读取固态硬盘的smartinfo中温度值,间隔1秒读一次 2、将获取的温度值按盘和时间点记录log文件中,log文件名用当前时间和测试盘来命名的 3、使用PyQt5将步骤(1)(2)集成到界面化:支持待测盘和测试时间自定义输入、硬盘的温度展示
时间: 2023-07-15 19:15:06 浏览: 232
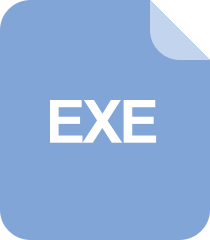
ssd测试工具
好的,这是一个测试sata ssd的python脚本,其中使用了PyQt5实现了界面化:
```python
import os
import time
import datetime
import subprocess
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QLineEdit
class SSDTest(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 待测盘标签和输入框
self.disk_label = QLabel('待测盘:', self)
self.disk_label.move(20, 20)
self.disk_input = QLineEdit(self)
self.disk_input.move(80, 20)
# 测试时间标签和输入框
self.time_label = QLabel('测试时间:', self)
self.time_label.move(20, 60)
self.time_input = QLineEdit(self)
self.time_input.move(80, 60)
# 开始测试按钮
self.start_button = QPushButton('开始测试', self)
self.start_button.move(20, 100)
self.start_button.clicked.connect(self.start_test)
# 温度展示标签
self.temp_label = QLabel('温度:', self)
self.temp_label.move(20, 140)
self.temp_value = QLabel('', self)
self.temp_value.move(80, 140)
self.setGeometry(300, 300, 250, 200)
self.setWindowTitle('SSD测试')
self.show()
def start_test(self):
# 获取待测盘和测试时间
disk = self.disk_input.text()
test_time = int(self.time_input.text())
# 获取当前时间并生成log文件名
now = datetime.datetime.now().strftime('%Y-%m-%d_%H-%M-%S')
log_file = f'{now}_{disk}.log'
# 打开log文件并写入表头
with open(log_file, 'w') as f:
f.write('时间\t温度\n')
# 读取温度并写入log文件
for i in range(test_time):
temp = self.get_temp(disk)
with open(log_file, 'a') as f:
f.write(f'{datetime.datetime.now()}\t{temp}\n')
self.temp_value.setText(str(temp)) # 更新温度展示
time.sleep(1)
def get_temp(self, disk):
# 读取smart信息
smart_info = subprocess.check_output(['smartctl', '-A', disk]).decode('utf-8').split('\n')
# 查找温度值
for line in smart_info:
if 'Temperature_Celsius' in line:
temp = line.split()[9]
return temp
if __name__ == '__main__':
app = QApplication([])
w = SSDTest()
app.exec_()
```
在运行脚本后,会出现一个界面,可以输入待测盘和测试时间,并且会实时展示当前硬盘的温度。点击“开始测试”按钮后,脚本会读取固态硬盘的smartinfo中温度值,并将获取的温度值按盘和时间点记录到log文件中,log文件名用当前时间和测试盘来命名的。
阅读全文
相关推荐
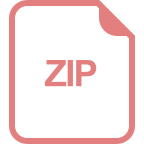
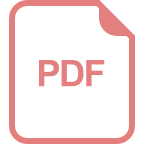





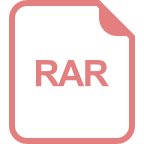
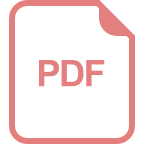
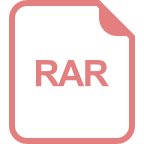
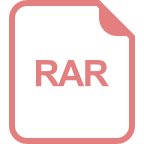