基于图像的苹果尺寸测量与坏果检测CNN代码
时间: 2023-06-15 21:05:54 浏览: 81
以下是一个基于图像的苹果尺寸测量与坏果检测的CNN代码:
```python
import os
import cv2
import numpy as np
import pandas as pd
import tensorflow as tf
from sklearn.model_selection import train_test_split
# 设置随机种子
np.random.seed(42)
tf.random.set_seed(42)
# 加载数据集
data_dir = "apple_dataset"
categories = os.listdir(data_dir)
labels = [i for i in range(len(categories))]
label_dict = dict(zip(categories, labels))
data = []
target = []
for category in categories:
folder_path = os.path.join(data_dir, category)
img_names = os.listdir(folder_path)
for img_name in img_names:
img_path = os.path.join(folder_path, img_name)
img = cv2.imread(img_path)
try:
# 将图像转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 将图像的大小调整为 100x100
resized = cv2.resize(gray, (100, 100))
# 将图像转换为数组
data.append(np.array(resized))
# 将图像的类别转换为数字
target.append(label_dict[category])
except Exception as e:
print('Exception:', e)
# 将数据和目标转换为 numpy 数组
data = np.array(data)/255.0
data = np.reshape(data, (data.shape[0], 100, 100, 1))
target = np.array(target)
# 将数据集划分为训练集和测试集
train_data, test_data, train_target, test_target = train_test_split(data, target, test_size=0.1)
# 创建 CNN 模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(200, (3,3), activation='relu', input_shape=(100, 100, 1)),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(100, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(50, activation='relu'),
tf.keras.layers.Dense(len(categories), activation='softmax')
])
# 编译模型
model.compile(loss='sparse_categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit(train_data, train_target, epochs=20, verbose=1)
# 评估模型
test_loss, test_acc = model.evaluate(test_data, test_target)
print("Test accuracy:", test_acc)
# 保存模型
model.save("apple_classification_model.h5")
```
这个代码将苹果数据集中的图像调整为 100x100 大小,并将其转换为灰度图。然后,它使用了一个简单的 CNN 模型来进行分类。这个模型包括两个卷积层和一个全连接层。最后,它使用了 `sparse_categorical_crossentropy` 损失函数和 `adam` 优化器进行训练。训练完成后,模型将保存到 `apple_classification_model.h5` 文件中。
相关推荐
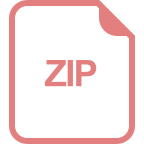














