public class IdiomProcessor { public static void main(String[] args) { // 定义输入文件路径和输出文件路径 String inputFile ="D:\yy\java\成语接龙\成语大全\去重后.txt"; String outputFile = "D:\yy\java\成语接龙\成语大全\全量成语处理后.txt"; try (BufferedReader reader = new BufferedReader(new FileReader(inputFile)); BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) { // 定义成语列表和成语末尾字与开头字相同的数量Map List<String> idiomList = new ArrayList<>(); Map<Character, Integer> countMap = new HashMap<>(); // 读取每一行成语信息 String line; while ((line = reader.readLine())!= null) { if (line.trim().isEmpty()) { continue; // 跳过空行 } if (line.contains("拼音:")) { // 处理包含指定内容的行 System.out.println("我动了"); // 将成语与其他信息分割 String[] parts = line.split("拼音:"); // 将成语加入成语列表 String idiom = parts[0]; idiomList.add(idiom); // 计算成语末尾字与开头字相同的数量 char lastChar = idiom.charAt(idiom.length() - 1); countMap.put(lastChar, countMap.getOrDefault(lastChar, 0) + 1); // 将原来的信息每一条加上count后输出到另一个文件 String newLine = line + "可接:" + countMap.get(lastChar) + "\n"; writer.write(newLine); } } } catch (IOException e) { e.printStackTrace(); } } }由于代码在用Map统计lastChar时不同成语的末尾字会相同,所以用Map记录时,输出的count有错,如何改正
时间: 2024-03-13 22:46:11 浏览: 161
可以使用一个 `Map<String, Integer>` 来记录每个成语的末尾字与开头字相同的数量。
具体的做法是,在计算成语末尾字与开头字相同的数量时,将 `countMap` 改为 `idiomCountMap`,并且将 key 从 `Character` 改为 `String`,其 value 记录的是成语末尾字与开头字相同的数量。
修改后的代码如下:
```
public class IdiomProcessor {
public static void main(String[] args) {
// 定义输入文件路径和输出文件路径
String inputFile = "D:\\yy\\java\\成语接龙\\成语大全\\去重后.txt";
String outputFile = "D:\\yy\\java\\成语接龙\\成语大全\\全量成语处理后.txt";
try (BufferedReader reader = new BufferedReader(new FileReader(inputFile));
BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) {
// 定义成语列表和成语末尾字与开头字相同的数量Map
List<String> idiomList = new ArrayList<>();
Map<String, Integer> idiomCountMap = new HashMap<>();
// 读取每一行成语信息
String line;
while ((line = reader.readLine()) != null) {
if (line.trim().isEmpty()) {
continue; // 跳过空行
}
if (line.contains("拼音:")) { // 处理包含指定内容的行
System.out.println("我动了");
// 将成语与其他信息分割
String[] parts = line.split("拼音:");
// 将成语加入成语列表
String idiom = parts[0];
idiomList.add(idiom);
// 计算成语末尾字与开头字相同的数量
char lastChar = idiom.charAt(idiom.length() - 1);
String key = lastChar + parts[1].substring(0, 1);
idiomCountMap.put(key, idiomCountMap.getOrDefault(key, 0) + 1);
// 将原来的信息每一条加上count后输出到另一个文件
String newLine = line + "可接:" + idiomCountMap.get(key) + "\n";
writer.write(newLine);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这样,就可以正确统计每个不同成语的末尾字与开头字相同的数量了。
阅读全文
相关推荐
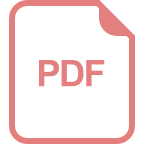
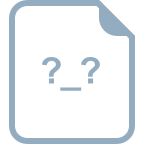
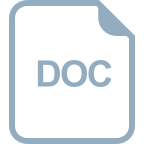
















