Python使用nums找最大值
时间: 2023-10-09 15:12:05 浏览: 69
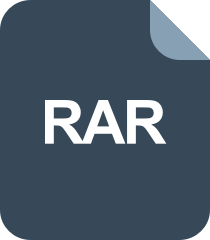
python找出最大子矩阵代码.rar
如果你想找到一个列表中的最大值,你可以使用Python内置函数`max()`。
例如,如果你有一个名为`nums`的列表,你可以使用以下代码找到它的最大值:
```
nums = [1, 3, 2, 5, 4]
max_num = max(nums)
print(max_num) # 输出:5
```
这将打印出`nums`列表中的最大值5。
阅读全文
相关推荐
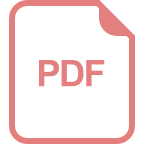
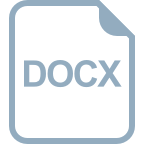















