LSTM预测python和matlab代码
时间: 2023-08-25 15:39:14 浏览: 57
以下是使用LSTM进行时间序列预测的Python代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from keras.models import Sequential
from keras.layers import Dense, LSTM
# 生成时间序列数据
def generate_data():
x = np.linspace(0, 10, 1000)
y = np.sin(x) + np.random.uniform(-0.5, 0.5, 1000)
return x, y
# 构建LSTM模型
def build_model():
model = Sequential()
model.add(LSTM(50, input_shape=(1, 1)))
model.add(Dense(1))
model.compile(loss='mse', optimizer='adam')
return model
# 训练模型
def train_model(model, x, y):
x = x.reshape(len(x), 1, 1)
model.fit(x, y, epochs=50, batch_size=32)
# 预测模型
def predict(model, x):
x = x.reshape(len(x), 1, 1)
y_pred = model.predict(x)
return y_pred.flatten()
# 绘制结果
def plot_result(x, y_true, y_pred):
plt.plot(x, y_true, label='True')
plt.plot(x, y_pred, label='Predicted')
plt.legend()
plt.show()
# 主函数
if __name__ == '__main__':
x, y_true = generate_data()
model = build_model()
train_model(model, x, y_true)
y_pred = predict(model, x)
plot_result(x, y_true, y_pred)
```
以下是使用LSTM进行时间序列预测的MATLAB代码:
```matlab
% 生成时间序列数据
t = linspace(0, 10, 1000);
y = sin(t) + 0.1 * randn(size(t));
% 构建LSTM模型
layers = [ ...
sequenceInputLayer(1)
lstmLayer(50)
fullyConnectedLayer(1)
regressionLayer];
options = trainingOptions(...
'adam', ...
'MaxEpochs', 50, ...
'MiniBatchSize', 32, ...
'Plots', 'training-progress');
net = trainNetwork(t', y', layers, options);
% 预测模型
y_pred = predict(net, t');
% 绘制结果
plot(t, y, t, y_pred)
legend('True', 'Predicted')
```
相关推荐
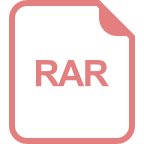
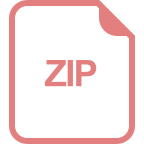














