# 指定文件夹并读取其中的数据 def read_data(folder, data_root="./work"): data = [] # 从train和valid文件夹分别读取有缺陷和无缺陷图像 if folder == 'train' or folder == 'valid': for label in ['defective', 'no_defective']: folder_name = os.path.join(data_root, folder, label) for file in tqdm(os.listdir(folder_name)): img = cv2.resize(cv2.imread(os.path.join(folder_name, file)), (224, 224)) # 令图像大小为224*224 data.append([img, 1 if label == 'defective' else 0]) # 有缺陷标注为1,无缺陷标注为0 if folder == 'train': # 对数据进行镜像处理以增加训练集数量 # 水平和垂直镜像 ############################################ img2 = img3 = data.append([img2, 1 if label == 'defective' else 0]) data.append([img3, 1 if label == 'defective' else 0]) # data.append([img4, 1 if label == 'defective' else 0]) ############################################ return data # 读取test文件夹全部数据 else: filepath = [] folder_name = os.path.join(data_root, folder) for file in tqdm(os.listdir(folder_name)): ################################################# ################################################# return data, filepath
时间: 2024-02-15 20:28:40 浏览: 99
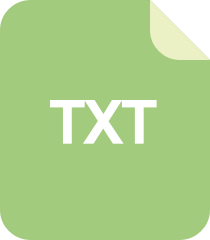
文件夹信息读取
这是一个 Python 函数 `read_data`,用于从指定文件夹中读取图像数据。其中:
- `folder` 表示要读取的文件夹名称,可以是 train、valid 或 test。
- `data_root` 表示数据所在的根目录,默认为 "./work"。
- 对于 train 和 valid 文件夹,函数会分别读取有缺陷和无缺陷的图像,并将它们的标签分别标注为 1 和 0。对于 train 数据还会进行数据增强,即对图像进行水平和垂直镜像,以增加训练集数量。
- 对于 test 文件夹,函数会读取全部数据,并返回数据和文件路径。
这段代码似乎存在缺陷,其中有两个变量 `img2` 和 `img3` 没有被定义,可能需要根据具体情况进行补充。
阅读全文
相关推荐
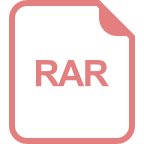
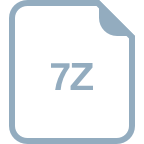




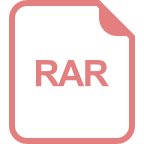
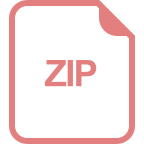
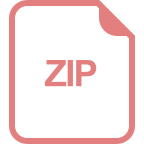
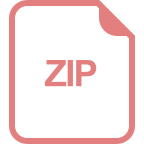
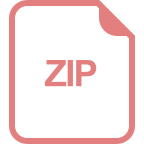
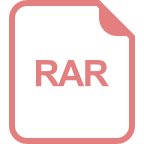
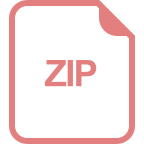
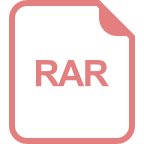
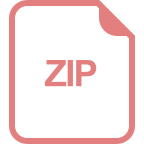
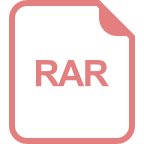
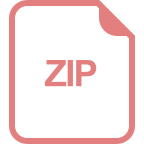
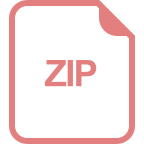