你用代码实现上面的思路
时间: 2024-02-25 13:56:26 浏览: 76
好的,以下是一个简单的 C++ 实现,用时间片轮转算法模拟进程调度:
```c++
#include <iostream>
#include <queue>
using namespace std;
// 进程控制块 (PCB)
struct PCB {
int pid; // 进程 ID
int priority; // 进程优先级
int burst_time; // 进程需要执行的时间
int remaining_time; // 进程剩余需要执行的时间
int wait_time; // 进程等待时间
};
// 进程比较函数,按照进程优先级从高到低排序
struct ComparePriority {
bool operator()(const PCB& p1, const PCB& p2) {
return p1.priority < p2.priority;
}
};
// 时间片轮转调度算法
void time_slice_round_robin(int time_slice, queue<PCB> &ready_queue) {
PCB running_process;
int time_remaining = time_slice;
while (!ready_queue.empty()) {
running_process = ready_queue.front();
ready_queue.pop();
// 模拟进程执行
while (running_process.remaining_time > 0 && time_remaining > 0) {
running_process.remaining_time--;
time_remaining--;
}
// 如果进程还没有执行完,则加入就绪队列末尾
if (running_process.remaining_time > 0) {
ready_queue.push(running_process);
} else {
// 进程执行完毕,输出进程信息
cout << "Process " << running_process.pid << " finished. "
<< "Waiting time: " << running_process.wait_time
<< ", Burst time: " << running_process.burst_time << endl;
}
// 时间片用完,将进程加入就绪队列末尾
if (time_remaining == 0) {
ready_queue.push(running_process);
time_remaining = time_slice;
}
}
}
int main() {
// 创建三个进程
PCB process1 = {1, 2, 10, 10, 0};
PCB process2 = {2, 1, 5, 5, 0};
PCB process3 = {3, 3, 7, 7, 0};
// 将进程加入就绪队列
queue<PCB> ready_queue;
ready_queue.push(process1);
ready_queue.push(process2);
ready_queue.push(process3);
// 执行时间片轮转调度算法,时间片为 2
time_slice_round_robin(2, ready_queue);
return 0;
}
```
以上代码只是一个简单的实现,可能还需要根据实际需求进行调整。希望能对你有所帮助!
阅读全文
相关推荐
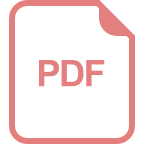


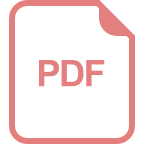
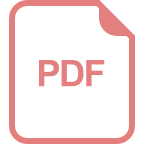
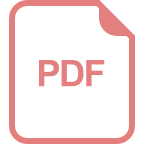
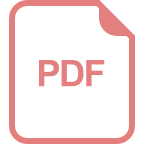








